mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-07-02 06:26:07 +02:00
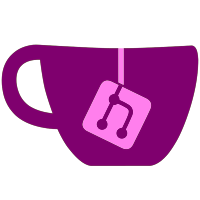
We do not want to allow saving of user data on unsigned builds as they don't have a stable signature and will not properly handle reinstallation. This can lead to a situation where the user has to resort to complex techniques to completely uninstall the package such as ADB or calling into PM directly.
147 lines
4.1 KiB
Groovy
147 lines
4.1 KiB
Groovy
plugins {
|
|
id 'com.android.application'
|
|
id 'kotlin-android'
|
|
id 'kotlin-kapt'
|
|
id 'dagger.hilt.android.plugin'
|
|
id 'idea'
|
|
id 'org.jetbrains.kotlin.plugin.serialization' version "$kotlin_version"
|
|
}
|
|
|
|
idea.module {
|
|
// These are not viable to index on most systems to exclude them to prevent IDE crashes
|
|
excludeDirs.add(file("libraries/boost"))
|
|
excludeDirs.add(file("libraries/llvm"))
|
|
}
|
|
|
|
android {
|
|
namespace 'emu.skyline'
|
|
|
|
var isBuildSigned = (System.getenv("CI") == "true") && (System.getenv("IS_SKYLINE_SIGNED") == "true")
|
|
|
|
compileSdkVersion 31
|
|
buildToolsVersion '33.0.0'
|
|
defaultConfig {
|
|
applicationId "skyline.emu"
|
|
|
|
minSdkVersion 29
|
|
targetSdkVersion 31
|
|
|
|
versionCode 3
|
|
versionName "0.0.3"
|
|
|
|
ndk {
|
|
abiFilters "arm64-v8a"
|
|
}
|
|
|
|
if (isBuildSigned)
|
|
manifestPlaceholders = [shouldSaveUserData: "true"]
|
|
else
|
|
manifestPlaceholders = [shouldSaveUserData: "false"]
|
|
}
|
|
|
|
/* JVM Bytecode Options */
|
|
def javaVersion = JavaVersion.VERSION_1_8
|
|
compileOptions {
|
|
sourceCompatibility = javaVersion
|
|
targetCompatibility = javaVersion
|
|
}
|
|
kotlinOptions {
|
|
jvmTarget = javaVersion.toString()
|
|
freeCompilerArgs += "-opt-in=kotlin.RequiresOptIn"
|
|
}
|
|
|
|
signingConfigs {
|
|
ci {
|
|
storeFile file(System.getenv("SIGNING_STORE_PATH") ?: "${System.getenv("user.home")}/keystore.jks")
|
|
storePassword System.getenv("SIGNING_STORE_PASSWORD")
|
|
keyAlias System.getenv("SIGNING_KEY_ALIAS")
|
|
keyPassword System.getenv("SIGNING_KEY_PASSWORD")
|
|
}
|
|
}
|
|
|
|
buildTypes {
|
|
release {
|
|
debuggable true
|
|
externalNativeBuild {
|
|
cmake {
|
|
arguments "-DCMAKE_BUILD_TYPE=RELEASE"
|
|
}
|
|
}
|
|
minifyEnabled true
|
|
shrinkResources true
|
|
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
|
|
signingConfig = isBuildSigned ? signingConfigs.ci : signingConfigs.debug
|
|
}
|
|
|
|
debug {
|
|
debuggable true
|
|
minifyEnabled false
|
|
shrinkResources false
|
|
signingConfig = isBuildSigned ? signingConfigs.ci : signingConfigs.debug
|
|
}
|
|
}
|
|
|
|
buildFeatures {
|
|
viewBinding true
|
|
}
|
|
|
|
/* Linting */
|
|
lint {
|
|
disable 'IconLocation'
|
|
}
|
|
|
|
/* NDK and CMake */
|
|
ndkVersion '25.0.8221429'
|
|
externalNativeBuild {
|
|
cmake {
|
|
version '3.18.1+'
|
|
path "CMakeLists.txt"
|
|
}
|
|
}
|
|
|
|
/* Android Assets */
|
|
androidResources {
|
|
ignoreAssetsPattern '*.md'
|
|
}
|
|
|
|
/* Vulkan Validation Layers */
|
|
sourceSets {
|
|
debug {
|
|
jniLibs {
|
|
srcDir "libraries/vklayers"
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
/* Google */
|
|
implementation 'androidx.core:core-ktx:1.7.0'
|
|
implementation 'androidx.appcompat:appcompat:1.4.1'
|
|
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
|
|
implementation 'androidx.preference:preference-ktx:1.2.0'
|
|
implementation 'com.google.android.material:material:1.5.0'
|
|
implementation 'androidx.documentfile:documentfile:1.0.1'
|
|
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0'
|
|
implementation "androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycle_version"
|
|
implementation "androidx.lifecycle:lifecycle-livedata-ktx:$lifecycle_version"
|
|
implementation 'androidx.fragment:fragment-ktx:1.4.1'
|
|
implementation "com.google.dagger:hilt-android:$hilt_version"
|
|
kapt "com.google.dagger:hilt-android-compiler:$hilt_version"
|
|
implementation 'com.google.android.flexbox:flexbox:3.0.0'
|
|
|
|
/* Kotlin */
|
|
implementation "org.jetbrains.kotlin:kotlin-reflect:$kotlin_version"
|
|
implementation "org.jetbrains.kotlinx:kotlinx-serialization-json:$serialization_version"
|
|
|
|
/* JetBrains */
|
|
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version"
|
|
|
|
/* Other Java */
|
|
implementation 'info.debatty:java-string-similarity:2.0.0'
|
|
}
|
|
|
|
kapt {
|
|
correctErrorTypes true
|
|
}
|