mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-11-15 16:05:10 +01:00
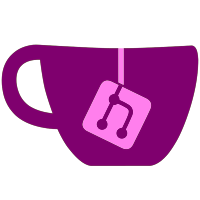
*Fixed freeze in theme menu when its empty *Optimized the game list loading on fat/ntfs/ext. This should speed up the loading process. *Added cache of game titles. This will speed up the startup after the cache file is written (in other words on second start of this rev). A TitlesCache.bin will be created in the same path as the wiitdb.xml for this. This should especial speed up the startup of fat/ntfs/ext partitions by a lot if no sub folders with the game titles are used, like GAMEID.wbfs only. That must have been painfully slow before on a lot of games. Should be at about the same speed as with sub folders now. I would still recommend to use sub folders. *Removed wiilight (disc slot) blinking when switching USB port on Hermes cIOSes (thanks rodries) *Added the ehcmodule sources from rodries to the branches *Updated language files
83 lines
2.6 KiB
C
83 lines
2.6 KiB
C
/*
|
|
* Original Copyright (c) 2001 by David Brownell
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
|
|
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software Foundation,
|
|
* Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
|
|
/* this file is part of ehci.c */
|
|
|
|
|
|
static inline void ehci_qtd_init(struct ehci_qtd *qtd
|
|
)
|
|
{
|
|
dma_addr_t dma = ehci_virt_to_dma(qtd);
|
|
memset (qtd, 0, sizeof *qtd);
|
|
qtd->qtd_dma = dma;
|
|
qtd->hw_token = (QTD_STS_HALT);
|
|
qtd->hw_next = EHCI_LIST_END();
|
|
qtd->hw_alt_next = EHCI_LIST_END();
|
|
}
|
|
|
|
int qtd_alt_mem=0; // double buffer qtd
|
|
|
|
static inline struct ehci_qtd * ehci_qtd_alloc(void)
|
|
{
|
|
struct ehci_qtd *qtd ;
|
|
//debug_printf("ehci_qtd used=%x\n",ehci->qtd_used);
|
|
if(ehci->qtd_used>=(EHCI_MAX_QTD)) return NULL;
|
|
//qtd = ehci->qtds[ehci->qtd_used++];
|
|
qtd = (void *) (((char *) ehci->qtds[0])+(ehci->qtd_used+EHCI_MAX_QTD*qtd_alt_mem)*((sizeof(struct ehci_qtd)+31) & ~31));
|
|
ehci->qtd_used++;
|
|
ehci_qtd_init(qtd);
|
|
return qtd;
|
|
}
|
|
|
|
|
|
|
|
|
|
|
|
int ehci_mem_init (void)
|
|
{
|
|
int i;
|
|
#if 1
|
|
ehci->periodic = ehci_maligned(DEFAULT_I_TDPS * sizeof(__le32),32,4096);
|
|
ehci->periodic_dma = ehci_virt_to_dma(ehci->periodic);
|
|
|
|
for (i = 0; i < DEFAULT_I_TDPS; i++)
|
|
ehci->periodic [i] = EHCI_LIST_END();
|
|
ehci_writel(ehci->periodic_dma, &ehci->regs->frame_list);
|
|
#else
|
|
debug_printf("ehci periodic:%x\n",ehci_readl(ehci, &ehci->regs->frame_list));
|
|
debug_printf("ehci *periodic:%x\n",*(u32*)ehci_readl(ehci, &ehci->regs->frame_list));
|
|
#endif
|
|
for(i=0;i<EHCI_MAX_QTD;i++)
|
|
ehci->qtds[i] = ehci_maligned(sizeof(struct ehci_qtd),(i==0) ? 4096 : 32,4096);
|
|
ehci->qtd_used = 0;
|
|
|
|
ehci->async = ehci_maligned(sizeof(struct ehci_qh),/*32*/4096,4096);
|
|
ehci->async->ehci = ehci;
|
|
ehci->async->qh_dma = ehci_virt_to_dma(ehci->async);
|
|
ehci->async->qtd_head = NULL;
|
|
|
|
ehci->asyncqh = ehci_maligned(sizeof(struct ehci_qh),32,4096);
|
|
ehci->asyncqh->ehci = ehci;
|
|
ehci->asyncqh->qh_dma = ehci_virt_to_dma(ehci->asyncqh);
|
|
ehci->asyncqh->qtd_head = NULL;
|
|
|
|
|
|
|
|
return 0;
|
|
}
|