mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-11 12:21:49 +02:00
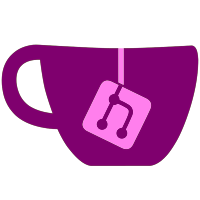
*Fixed freeze in theme menu when its empty *Optimized the game list loading on fat/ntfs/ext. This should speed up the loading process. *Added cache of game titles. This will speed up the startup after the cache file is written (in other words on second start of this rev). A TitlesCache.bin will be created in the same path as the wiitdb.xml for this. This should especial speed up the startup of fat/ntfs/ext partitions by a lot if no sub folders with the game titles are used, like GAMEID.wbfs only. That must have been painfully slow before on a lot of games. Should be at about the same speed as with sub folders now. I would still recommend to use sub folders. *Removed wiilight (disc slot) blinking when switching USB port on Hermes cIOSes (thanks rodries) *Added the ehcmodule sources from rodries to the branches *Updated language files
52 lines
1.4 KiB
C++
52 lines
1.4 KiB
C++
#ifndef WIITDB_TITLES_H_
|
|
#define WIITDB_TITLES_H_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <gctypes.h>
|
|
#include "usbloader/disc.h"
|
|
|
|
typedef struct _GameTitle
|
|
{
|
|
char GameID[7];
|
|
std::string Title;
|
|
int ParentalRating;
|
|
int PlayersCount;
|
|
|
|
} GameTitle;
|
|
|
|
class CGameTitles
|
|
{
|
|
public:
|
|
//! Set a game title from wiitdb
|
|
void SetGameTitle(const char * id, const char * title);
|
|
//! Overload
|
|
void SetGameTitle(const u8 * id, const char * title) { SetGameTitle((const char *) id, title); };
|
|
|
|
//! Get a game title
|
|
const char * GetTitle(const char * id) const;
|
|
//! Overload
|
|
const char * GetTitle(const u8 * id) const { return GetTitle((const char *) id); };
|
|
//! Overload
|
|
const char * GetTitle(const struct discHdr *header) const;
|
|
|
|
//! Get game parental rating
|
|
int GetParentalRating(const char * id) const;
|
|
//! Get possible number of players for this game
|
|
int GetPlayersCount(const char * id) const;
|
|
//! Load Game Titles from WiiTDB
|
|
void LoadTitlesFromWiiTDB(const char * path);
|
|
//! Set default game titles
|
|
void SetDefault();
|
|
protected:
|
|
u32 ReadCachedTitles(const char * path);
|
|
void WriteCachedTitles(const char * path);
|
|
void RemoveUnusedCache(std::vector<std::string> &MissingTitles);
|
|
|
|
std::vector<GameTitle> TitleList;
|
|
};
|
|
|
|
extern CGameTitles GameTitles;
|
|
|
|
#endif
|