mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-11-15 16:05:10 +01:00
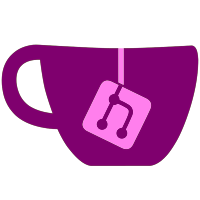
*Fixed freeze in theme menu when its empty *Optimized the game list loading on fat/ntfs/ext. This should speed up the loading process. *Added cache of game titles. This will speed up the startup after the cache file is written (in other words on second start of this rev). A TitlesCache.bin will be created in the same path as the wiitdb.xml for this. This should especial speed up the startup of fat/ntfs/ext partitions by a lot if no sub folders with the game titles are used, like GAMEID.wbfs only. That must have been painfully slow before on a lot of games. Should be at about the same speed as with sub folders now. I would still recommend to use sub folders. *Removed wiilight (disc slot) blinking when switching USB port on Hermes cIOSes (thanks rodries) *Added the ehcmodule sources from rodries to the branches *Updated language files
61 lines
1.6 KiB
C
61 lines
1.6 KiB
C
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <malloc.h>
|
|
#include <ogcsys.h>
|
|
#include <gccore.h>
|
|
|
|
//#define OH0_REPL
|
|
#ifdef OH0_REPL
|
|
#include "oh0_elf.h"
|
|
#endif
|
|
struct ios_module_replacement
|
|
{
|
|
char *name;
|
|
u8 *data;
|
|
u32 *size;
|
|
};
|
|
|
|
struct ios_module_replacement ios_repls[]={
|
|
#ifdef OH0_REPL
|
|
{"OH0",(u8*)oh0_elf,(u32*)&oh0_elf_size}
|
|
#endif
|
|
};
|
|
|
|
void debug_printf(const char *fmt, ...);
|
|
|
|
int replace_ios_modules(u8 **decrypted_buf, u32 *content_size)
|
|
{
|
|
int i,version;
|
|
u32 len;
|
|
u8*buf = *decrypted_buf;
|
|
char *ios_version_tag = "$IOSVersion:";
|
|
len = *content_size;
|
|
if (len == 64)
|
|
return 0;
|
|
version =0;
|
|
for (i=0; i<(len-64); i++)
|
|
if (!strncmp((char *)buf+i, ios_version_tag, 10)) {
|
|
version = i;
|
|
break;
|
|
}
|
|
|
|
for (i=0;i<sizeof(ios_repls)/sizeof(ios_repls[0]); i++){
|
|
struct ios_module_replacement *repl = &ios_repls[i];
|
|
if(!memcmp(buf+version+13,repl->name,strlen(repl->name)))
|
|
{
|
|
debug_printf("replaced %s\n",repl->name);
|
|
free(*decrypted_buf);
|
|
len = *repl->size;
|
|
len+=31;
|
|
len&=~31;
|
|
*decrypted_buf = malloc(len);
|
|
memcpy(*decrypted_buf,repl->data,len);
|
|
*content_size = len;
|
|
return 1;
|
|
}
|
|
}
|
|
return 0;
|
|
}
|
|
|