mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-10 20:01:50 +02:00
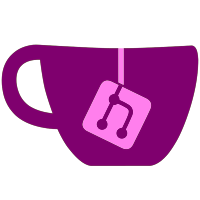
formatted the code to make it easier to read. no functional changes at all. i didn't put anything from the libwiigui folder or banner folder in the beautifier. my automated .bat seems to have done a good job. the only places i see it fucked up was on (GXColor){blablabla}. it treated the brackets in the color like all the other brackets and put the color on a new line and indented it. i think i fixed most of them. not sure if it messed up anywhere else. also not sure about how it handled different linebreaks. it looks fine on windows. if it looks messed up on linux, it can be reverted. the code still compiles and runs fine.
241 lines
6.6 KiB
C
241 lines
6.6 KiB
C
/*
|
|
miniunz.c
|
|
Version 1.01e, February 12th, 2005
|
|
|
|
Copyright (C) 1998-2005 Gilles Vollant
|
|
*/
|
|
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <time.h>
|
|
#include <errno.h>
|
|
#include <fcntl.h>
|
|
# include <unistd.h>
|
|
# include <utime.h>
|
|
|
|
#include "unzip.h"
|
|
|
|
#define CASESENSITIVITY (0)
|
|
#define WRITEBUFFERSIZE (8192)
|
|
#define MAXFILENAME (256)
|
|
|
|
static int mymkdir(const char* dirname) {
|
|
int ret=0;
|
|
ret = mkdir (dirname,0775);
|
|
return ret;
|
|
}
|
|
|
|
int makedir (char *newdir) {
|
|
char *buffer ;
|
|
char *p;
|
|
int len = (int)strlen(newdir);
|
|
|
|
if (len <= 0)
|
|
return 0;
|
|
|
|
buffer = (char*)malloc(len+1);
|
|
strcpy(buffer,newdir);
|
|
|
|
if (buffer[len-1] == '/') {
|
|
buffer[len-1] = '\0';
|
|
}
|
|
if (mymkdir(buffer) == 0) {
|
|
free(buffer);
|
|
return 1;
|
|
}
|
|
|
|
p = buffer+1;
|
|
while (1) {
|
|
char hold;
|
|
|
|
while (*p && *p != '\\' && *p != '/')
|
|
p++;
|
|
hold = *p;
|
|
*p = 0;
|
|
if ((mymkdir(buffer) == -1) && (errno == ENOENT)) {
|
|
printf("couldn't create directory %s\n",buffer);
|
|
free(buffer);
|
|
return 0;
|
|
}
|
|
if (hold == 0)
|
|
break;
|
|
*p++ = hold;
|
|
}
|
|
free(buffer);
|
|
return 1;
|
|
}
|
|
|
|
static int do_extract_currentfile(unzFile uf,const int* popt_extract_without_path,int* popt_overwrite,const char* password) {
|
|
char filename_inzip[256];
|
|
char* filename_withoutpath;
|
|
char* p;
|
|
int err=UNZ_OK;
|
|
FILE *fout=NULL;
|
|
void* buf;
|
|
uInt size_buf;
|
|
|
|
unz_file_info file_info;
|
|
err = unzGetCurrentFileInfo(uf,&file_info,filename_inzip,sizeof(filename_inzip),NULL,0,NULL,0);
|
|
|
|
if (err!=UNZ_OK) {
|
|
printf("error %d with zipfile in unzGetCurrentFileInfo\n",err);
|
|
return err;
|
|
}
|
|
|
|
size_buf = WRITEBUFFERSIZE;
|
|
buf = (void*)malloc(size_buf);
|
|
if (buf==NULL) {
|
|
printf("Error allocating memory\n");
|
|
return UNZ_INTERNALERROR;
|
|
}
|
|
|
|
p = filename_withoutpath = filename_inzip;
|
|
while ((*p) != '\0') {
|
|
if (((*p)=='/') || ((*p)=='\\'))
|
|
filename_withoutpath = p+1;
|
|
p++;
|
|
}
|
|
|
|
if ((*filename_withoutpath)=='\0') {
|
|
if ((*popt_extract_without_path)==0) {
|
|
printf("creating directory: %s\n",filename_inzip);
|
|
mymkdir(filename_inzip);
|
|
}
|
|
} else {
|
|
char* write_filename;
|
|
int skip=0;
|
|
|
|
if ((*popt_extract_without_path)==0)
|
|
write_filename = filename_inzip;
|
|
else
|
|
write_filename = filename_withoutpath;
|
|
|
|
err = unzOpenCurrentFilePassword(uf,password);
|
|
if (err!=UNZ_OK) {
|
|
printf("error %d with zipfile in unzOpenCurrentFilePassword\n",err);
|
|
}
|
|
|
|
if (((*popt_overwrite)==0) && (err==UNZ_OK)) {
|
|
char rep=0;
|
|
FILE* ftestexist;
|
|
ftestexist = fopen(write_filename,"rb");
|
|
if (ftestexist!=NULL) {
|
|
fclose(ftestexist);
|
|
do {
|
|
char answer[128];
|
|
int ret;
|
|
|
|
printf("The file %s exists. Overwrite ? [y]es, [n]o, [A]ll: ",write_filename);
|
|
ret = scanf("%1s",answer);
|
|
if (ret != 1) {
|
|
exit(EXIT_FAILURE);
|
|
}
|
|
rep = answer[0] ;
|
|
if ((rep>='a') && (rep<='z'))
|
|
rep -= 0x20;
|
|
} while ((rep!='Y') && (rep!='N') && (rep!='A'));
|
|
}
|
|
|
|
if (rep == 'N')
|
|
skip = 1;
|
|
|
|
if (rep == 'A')
|
|
*popt_overwrite=1;
|
|
}
|
|
|
|
if ((skip==0) && (err==UNZ_OK)) {
|
|
fout=fopen(write_filename,"wb");
|
|
|
|
/* some zipfile don't contain directory alone before file */
|
|
if ((fout==NULL) && ((*popt_extract_without_path)==0) &&
|
|
(filename_withoutpath!=(char*)filename_inzip)) {
|
|
char c=*(filename_withoutpath-1);
|
|
*(filename_withoutpath-1)='\0';
|
|
makedir(write_filename);
|
|
*(filename_withoutpath-1)=c;
|
|
fout=fopen(write_filename,"wb");
|
|
}
|
|
|
|
if (fout==NULL) {
|
|
printf("error opening %s\n",write_filename);
|
|
}
|
|
}
|
|
|
|
if (fout!=NULL) {
|
|
printf(" extracting: %s\n",write_filename);
|
|
|
|
do {
|
|
err = unzReadCurrentFile(uf,buf,size_buf);
|
|
if (err<0) {
|
|
printf("error %d with zipfile in unzReadCurrentFile\n",err);
|
|
break;
|
|
}
|
|
if (err>0)
|
|
if (fwrite(buf,err,1,fout)!=1) {
|
|
printf("error in writing extracted file\n");
|
|
err=UNZ_ERRNO;
|
|
break;
|
|
}
|
|
} while (err>0);
|
|
if (fout)
|
|
fclose(fout);
|
|
|
|
}
|
|
|
|
if (err==UNZ_OK) {
|
|
err = unzCloseCurrentFile (uf);
|
|
if (err!=UNZ_OK) {
|
|
printf("error %d with zipfile in unzCloseCurrentFile\n",err);
|
|
}
|
|
} else
|
|
unzCloseCurrentFile(uf); /* don't lose the error */
|
|
}
|
|
|
|
free(buf);
|
|
return err;
|
|
}
|
|
|
|
|
|
int extractZip(unzFile uf,int opt_extract_without_path,int opt_overwrite,const char* password) {
|
|
uLong i;
|
|
unz_global_info gi;
|
|
int err;
|
|
|
|
err = unzGetGlobalInfo (uf,&gi);
|
|
if (err!=UNZ_OK)
|
|
printf("error %d with zipfile in unzGetGlobalInfo \n",err);
|
|
|
|
for (i=0;i<gi.number_entry;i++) {
|
|
if (do_extract_currentfile(uf,&opt_extract_without_path,
|
|
&opt_overwrite,
|
|
password) != UNZ_OK)
|
|
break;
|
|
|
|
if ((i+1)<gi.number_entry) {
|
|
err = unzGoToNextFile(uf);
|
|
if (err!=UNZ_OK) {
|
|
printf("error %d with zipfile in unzGoToNextFile\n",err);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
int extractZipOnefile(unzFile uf,const char* filename,int opt_extract_without_path,int opt_overwrite,const char* password) {
|
|
if (unzLocateFile(uf,filename,CASESENSITIVITY)!=UNZ_OK) {
|
|
printf("file %s not found in the zipfile\n",filename);
|
|
return 2;
|
|
}
|
|
|
|
if (do_extract_currentfile(uf,&opt_extract_without_path,
|
|
&opt_overwrite,
|
|
password) == UNZ_OK)
|
|
return 0;
|
|
else
|
|
return 1;
|
|
}
|