mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-11-18 09:19:17 +01:00
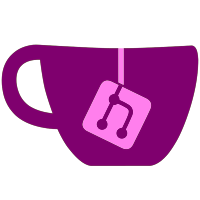
*Moved all related global settings to a settings class. one for themes and individual games will follow. Probably broke some settings or theme loading, we can deal with that later and fix when someone discovers bugs.
70 lines
2.3 KiB
C
70 lines
2.3 KiB
C
|
|
#ifndef _XML_H_
|
|
#define _XML_H_
|
|
|
|
#include <mxml.h>
|
|
|
|
|
|
// open database, close database, load info for a game
|
|
bool OpenXMLDatabase( char* xmlfilepath, char* argdblang, bool argJPtoEN, bool openfile, bool loadtitles, bool keepopen );
|
|
void CloseXMLDatabase();
|
|
bool LoadGameInfoFromXML( char* gameid, char* langcode );
|
|
|
|
#define XML_ELEMMAX 15
|
|
#define XML_SYNOPSISLEN 4000
|
|
|
|
struct gameXMLinfo
|
|
{
|
|
char id[7];
|
|
char version[50];
|
|
char region[7];
|
|
char title[200];
|
|
char synopsis[XML_SYNOPSISLEN];
|
|
char title_EN[200];
|
|
char synopsis_EN[XML_SYNOPSISLEN];
|
|
char locales[XML_ELEMMAX+1][5];
|
|
int localeCnt;
|
|
char developer[75];
|
|
char publisher[75];
|
|
char publisherfromid[75];
|
|
char year[5];
|
|
char month[3];
|
|
char day[3];
|
|
char genre[75];
|
|
char genresplit[XML_ELEMMAX+1][20];
|
|
int genreCnt;
|
|
char ratingtype[5];
|
|
char ratingvalue[5];
|
|
char ratingdescriptors[XML_ELEMMAX+1][40];
|
|
int descriptorCnt;
|
|
char ratingvalueCERO[5];
|
|
char ratingvalueESRB[5];
|
|
char ratingvaluePEGI[5];
|
|
char wifiplayers[4];
|
|
char wififeatures[XML_ELEMMAX+1][20];
|
|
int wifiCnt;
|
|
char players[4];
|
|
char accessories[XML_ELEMMAX+1][20];
|
|
int accessoryCnt;
|
|
char accessoriesReq[XML_ELEMMAX+1][20];
|
|
int accessoryReqCnt;
|
|
char iso_crc[9];
|
|
char iso_md5[33];
|
|
char iso_sha1[41];
|
|
} ;
|
|
|
|
bool OpenXMLFile( char* filename );
|
|
void LoadTitlesFromXML( char *langcode, bool forcejptoen );
|
|
void GetPublisherFromGameid( char *idtxt, char *dest, int destsize );
|
|
const char *ConvertLangTextToCode( char *langtext );
|
|
void ConvertRating( char *ratingvalue, char *fromrating, const char *torating, char *destvalue, int destsize );
|
|
void PrintGameInfo( bool showfullinfo );
|
|
char *MemInfo();
|
|
void GetTextFromNode( mxml_node_t *currentnode, mxml_node_t *topnode, const char *nodename,
|
|
const char *attributename, char *value, int descend, char *dest, int destsize );
|
|
int GetRatingForGame( char *gameid );
|
|
char * get_nodetext( mxml_node_t *node, char *buffer, int buflen );
|
|
|
|
#endif
|
|
|