mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-04 00:46:10 +02:00
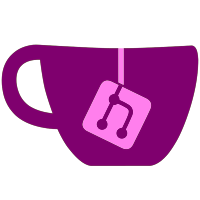
*WiiTDB now falls back to english if the locale language is not found for Titles/Synopsis *Some clean up and memory leak fixes in game info prompt and adjusted the info get to the new WiiTDB reading method. *Added a few new useful functions from WiiXplorer (DownloadToFileWithProgress, ShowError,...) *Disabled the console output after the GUI is started up. The info is only output to gecko in GUI mode now.
151 lines
6.2 KiB
C++
151 lines
6.2 KiB
C++
/****************************************************************************
|
|
* Copyright (C) 2010
|
|
* by Dimok
|
|
*
|
|
* This software is provided 'as-is', without any express or implied
|
|
* warranty. In no event will the authors be held liable for any
|
|
* damages arising from the use of this software.
|
|
*
|
|
* Permission is granted to anyone to use this software for any
|
|
* purpose, including commercial applications, and to alter it and
|
|
* redistribute it freely, subject to the following restrictions:
|
|
*
|
|
* 1. The origin of this software must not be misrepresented; you
|
|
* must not claim that you wrote the original software. If you use
|
|
* this software in a product, an acknowledgment in the product
|
|
* documentation would be appreciated but is not required.
|
|
*
|
|
* 2. Altered source versions must be plainly marked as such, and
|
|
* must not be misrepresented as being the original software.
|
|
*
|
|
* 3. This notice may not be removed or altered from any source
|
|
* distribution.
|
|
***************************************************************************/
|
|
#ifndef WIITDB_HPP_
|
|
#define WIITDB_HPP_
|
|
|
|
#include <vector>
|
|
#include <string>
|
|
|
|
using namespace std;
|
|
|
|
typedef struct _Accessoir
|
|
{
|
|
string Name;
|
|
bool Required;
|
|
} Accessoir;
|
|
|
|
typedef struct _GameXMLInfo
|
|
{
|
|
string GameID;
|
|
string Region;
|
|
string Title;
|
|
string Synopsis;
|
|
string Developer;
|
|
string Publisher;
|
|
unsigned int PublishDate;
|
|
vector<string> GenreList;
|
|
int RatingType;
|
|
string RatingValue;
|
|
vector<string> RatingDescriptorList;
|
|
int WifiPlayers;
|
|
vector<string> WifiFeatureList;
|
|
int Players;
|
|
vector<Accessoir> AccessoirList;
|
|
long CaseColor;
|
|
|
|
} GameXMLInfo;
|
|
|
|
typedef struct _GameOffsets
|
|
{
|
|
char gameID[7];
|
|
unsigned int gamenode;
|
|
unsigned int nodesize;
|
|
} __attribute__((__packed__)) GameOffsets;
|
|
|
|
class WiiTDB
|
|
{
|
|
public:
|
|
//! Constructor
|
|
WiiTDB();
|
|
//! Constructor
|
|
//! If filepath is passed the xml file is opened and the node offsets are loaded
|
|
WiiTDB(const char * filepath);
|
|
//! Destructor
|
|
~WiiTDB();
|
|
//! If filepath is passed the xml file is opened and the node offsets are loaded
|
|
bool OpenFile(const char * filepath);
|
|
//! Closes the WiiTDB xml file
|
|
void CloseFile();
|
|
//! Set the language code which should be use to find the appropriate language
|
|
//! If the language code is not found, the language code defaults to EN
|
|
void SetLanguageCode(const char * code) { if(code) LangCode = code; };
|
|
//! Get the current set language code
|
|
const char * GetLanguageCode() { return LangCode.c_str(); };
|
|
//! Get the title of a specific game id in the language defined in LangCode
|
|
bool GetTitle(const char * id, string & title);
|
|
//! Get the synopsis of a specific game id in the language defined in LangCode
|
|
bool GetSynopsis(const char * id, string & synopsis);
|
|
//! Get the region of a game for a specific game id
|
|
bool GetRegion(const char * id, string & region);
|
|
//! Get the developer of a game for a specific game id
|
|
bool GetDeveloper(const char * id, string & dev);
|
|
//! Get the publisher of a game for a specific game id
|
|
bool GetPublisher(const char * id, string & pub);
|
|
//! Get the publish date of a game for a specific game id
|
|
//! First 1 byte is the day, than 1 byte month and last 2 bytes is the year
|
|
//! year = (return >> 16), month = (return >> 8) & 0xFF, day = return & 0xFF
|
|
unsigned int GetPublishDate(const char * id);
|
|
//! Get the genre list of a game for a specific game id
|
|
bool GetGenreList(const char * id, vector<string> & genre);
|
|
//! Get the rating type for a specific game id
|
|
//! The rating type can be converted to a string with WiiTDB::RatingToString(rating)
|
|
int GetRating(const char * id);
|
|
//! Get the rating value for a specific game id
|
|
bool GetRatingValue(const char * id, string & rating_value);
|
|
//! Get the rating descriptor list inside a vector for a specific game id
|
|
//! Returns the amount of descriptors found or -1 if failed
|
|
int GetRatingDescriptorList(const char * id, vector<string> & desc_list);
|
|
//! Get the wifi player count for a specific game id
|
|
//! Returns the amount of wifi players or -1 if failed
|
|
int GetWifiPlayers(const char * id);
|
|
//! Get the wifi feature list inside a vector for a specific game id
|
|
//! Returns the amount of wifi features found or -1 if failed
|
|
int GetWifiFeatureList(const char * id, vector<string> & feat_list);
|
|
//! Get the player count for a specific game id
|
|
//! Returns the amount of players or -1 if failed
|
|
int GetPlayers(const char * id);
|
|
//! Returns the amount of accessoirs found or -1 if failed
|
|
//! Get the accessoir (inputs) list inside a vector for a specific game id
|
|
int GetAccessoirList(const char * id, vector<Accessoir> & acc_list);
|
|
//! Get the box (case) color for a specific game id
|
|
//! Returns the color in RGB (first 3 bytes)
|
|
int GetCaseColor(const char * id);
|
|
//! Get the complete game info in the GameXMLInfo struct
|
|
bool GetGameXMLInfo(const char * id, GameXMLInfo * gameInfo);
|
|
//! Convert a specific game rating to a string
|
|
static const char * RatingToString(int rating);
|
|
//! Get the version of the wiitdb xml database
|
|
unsigned long long GetWiiTDBVersion();
|
|
//! Get the entry count in the xml database
|
|
inline size_t GetEntryCount() { return OffsetMap.size(); };
|
|
private:
|
|
bool ParseFile();
|
|
bool LoadGameOffsets(const char * path);
|
|
bool SaveGameOffsets(const char * path);
|
|
inline size_t GetData(char * data, int offset, int size);
|
|
inline char * LoadGameNode(const char * id);
|
|
inline char * GetGameNode(const char * id);
|
|
inline GameOffsets * GetGameOffset(const char * id);
|
|
inline char * SeekLang(char * text, const char * langcode);
|
|
inline char * GetNodeText(char * data, const char * nodestart, const char * nodeend);
|
|
|
|
vector<GameOffsets> OffsetMap;
|
|
FILE * file;
|
|
string LangCode;
|
|
char * GameNodeCache;
|
|
char GameIDCache[7];
|
|
};
|
|
|
|
#endif
|