mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-12 04:41:49 +02:00
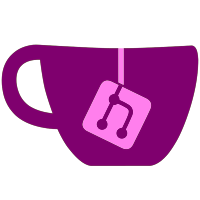
All options pages now contain the correct number of lines, which together with another adjustment should fix scrollbar alignment (issue 971). Added missing scrollbar on custom paths page. dimok fixed the file browser bug that prevented going back to the parent folder if the current folder was empty (issue 836). Fixed parental control keyboard bug introduced in r750 (issue 976). The free space text is now in correct order when the GUI is set to Japanese.
92 lines
2.2 KiB
C++
92 lines
2.2 KiB
C++
#include "gui.h"
|
|
|
|
class customOptionList {
|
|
public:
|
|
customOptionList(int size);
|
|
~customOptionList();
|
|
void SetLength(int size);
|
|
void SetName(int i, const char *format, ...) __attribute__((format (printf, 3, 4)));
|
|
const char *GetName(int i)
|
|
{
|
|
if(i >= 0 && i < length && name[i])
|
|
return name[i];
|
|
else
|
|
return "";
|
|
}
|
|
void SetValue(int i, const char *format, ...) __attribute__((format (printf, 3, 4)));
|
|
const char *GetValue(int i)
|
|
{
|
|
if(i >= 0 && i < length && value[i])
|
|
return value[i];
|
|
else
|
|
return "";
|
|
}
|
|
int GetLength() { return length; }
|
|
bool IsChanged() { bool ret = changed; changed = false; return ret;}
|
|
private:
|
|
int length;
|
|
char ** name;
|
|
char ** value;
|
|
bool changed;
|
|
};
|
|
|
|
//!Display a list of menu options
|
|
class GuiCustomOptionBrowser : public GuiElement
|
|
{
|
|
public:
|
|
GuiCustomOptionBrowser(int w, int h, customOptionList * l, const char * themePath, const char *custombg, const u8 *imagebg, int scrollbar, int col2);
|
|
~GuiCustomOptionBrowser();
|
|
int FindMenuItem(int c, int d);
|
|
int GetClickedOption();
|
|
int GetSelectedOption();
|
|
void SetClickable(bool enable);
|
|
void SetScrollbar(int enable);
|
|
void SetOffset(int optionnumber);
|
|
void ResetState();
|
|
void SetFocus(int f);
|
|
void Draw();
|
|
void Update(GuiTrigger * t);
|
|
protected:
|
|
void UpdateListEntries();
|
|
int selectedItem;
|
|
int listOffset;
|
|
int size;
|
|
int coL2;
|
|
int scrollbaron;
|
|
|
|
customOptionList * options;
|
|
int * optionIndex;
|
|
GuiButton ** optionBtn;
|
|
GuiText ** optionTxt;
|
|
GuiText ** optionVal;
|
|
GuiText ** optionValOver;
|
|
GuiImage ** optionBg;
|
|
|
|
GuiButton * arrowUpBtn;
|
|
GuiButton * arrowDownBtn;
|
|
GuiButton * scrollbarBoxBtn;
|
|
|
|
GuiImage * bgOptionsImg;
|
|
GuiImage * scrollbarImg;
|
|
GuiImage * arrowDownImg;
|
|
GuiImage * arrowDownOverImg;
|
|
GuiImage * arrowUpImg;
|
|
GuiImage * arrowUpOverImg;
|
|
GuiImage * scrollbarBoxImg;
|
|
GuiImage * scrollbarBoxOverImg;
|
|
|
|
GuiImageData * bgOptions;
|
|
GuiImageData * bgOptionsEntry;
|
|
GuiImageData * scrollbar;
|
|
GuiImageData * arrowDown;
|
|
GuiImageData * arrowDownOver;
|
|
GuiImageData * arrowUp;
|
|
GuiImageData * arrowUpOver;
|
|
GuiImageData * scrollbarBox;
|
|
GuiImageData * scrollbarBoxOver;
|
|
|
|
GuiSound * btnSoundClick;
|
|
GuiTrigger * trigA;
|
|
GuiTrigger * trigHeldA;
|
|
};
|