mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-02 07:56:09 +02:00
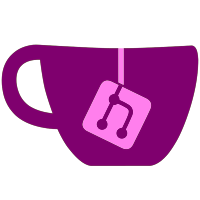
*Added Free Space show option to the settings (can be enabled even on FAT partitions) *Moved partition free space determination to a ThreadedTask because it takes up to 10 seconds on FAT. This speeds up the build up of the main game menu (on start and on change from other menu) on all partitions. *Removed double "waiting for slow HDD" screens *Fixed scrollbar in settings
29 lines
780 B
C++
29 lines
780 B
C++
#ifndef THREADED_TASK_HPP_
|
|
#define THREADED_TASK_HPP_
|
|
|
|
#include <gccore.h>
|
|
#include <vector>
|
|
#include "Callback.hpp"
|
|
|
|
class ThreadedTask
|
|
{
|
|
public:
|
|
static ThreadedTask * Instance() { if(!instance) instance = new ThreadedTask(); return instance; };
|
|
static void DestroyInstance() { delete instance; instance = NULL; };
|
|
void AddCallback(cCallback * c, void * arg = 0) { CallbackList.push_back(c); ArgList.push_back(arg); };
|
|
void Execute() { LWP_ResumeThread(Thread); };
|
|
private:
|
|
ThreadedTask();
|
|
~ThreadedTask();
|
|
void ThreadLoop(void *arg);
|
|
static void * ThreadCallback(void *arg);
|
|
|
|
static ThreadedTask *instance;
|
|
lwp_t Thread;
|
|
bool ExitRequested;
|
|
std::vector<cCallback *> CallbackList;
|
|
std::vector<void *> ArgList;
|
|
};
|
|
|
|
#endif
|