mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-10-02 03:35:09 +02:00
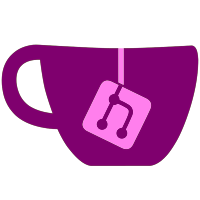
*Created an own class for the homebrew prompt *Created scrollbar class which is now used on every browser *Created a checkbox browser list class *Changed the category prompts to the new list mode *Improved B-Button scrolling *Fixed horizontal text scrolling *Fixed possible crash on long text display *Many internal gui changes and navigation changes *Fixed booting games by argument (headless id) (Issue 1930) *Fixed SD Reload button to really reload the SD after it was ejected (Issue 1923) *Added booting with arguements from meta.xml for homebrews (Issue 1926) *Added some arguments acception from meta.xml to our app. "-ios=xxx" and "-usbport=x" or "--ios=xxx" and "--usbport=x" can be used. -usbport is for Hermes cIOS to decide which usb port to use on startup. The ios is the boot IOS on startup, it always overrides the compiled boot IOS into the application.
70 lines
1.9 KiB
C++
70 lines
1.9 KiB
C++
#ifndef _TEXT_HPP_
|
|
#define _TEXT_HPP_
|
|
|
|
#include "GUI/gui.h"
|
|
#include "wstring.hpp"
|
|
|
|
typedef struct
|
|
{
|
|
int LineOffset;
|
|
int CharCount;
|
|
int width;
|
|
} TextLine;
|
|
|
|
class Text: public GuiText
|
|
{
|
|
public:
|
|
//!Constructor
|
|
//!\param t Text
|
|
//!\param s Font size
|
|
//!\param c Font color
|
|
Text(const char * t, int s, GXColor c);
|
|
Text(const wchar_t * t, int s, GXColor c);
|
|
~Text();
|
|
//!Sets the text of the GuiText element
|
|
//!\param t Text
|
|
void SetText(const char * t);
|
|
void SetText(const wchar_t * t);
|
|
//!Set the max texwidth
|
|
void SetMaxWidth(int width);
|
|
//!Go to next line
|
|
void NextLine();
|
|
//!Go to previous line
|
|
void PreviousLine();
|
|
//!Refresh the rows to draw
|
|
void Refresh();
|
|
//!Set the text line
|
|
void SetTextLine(int line);
|
|
//!Set to the char pos in text
|
|
void SetTextPos(int pos);
|
|
//!Refresh the rows to draw
|
|
int GetCurrPos() { return curLineStart; };
|
|
//!Get the count of loaded lines
|
|
int GetLinesCount() { return textDyn.size(); };
|
|
//!Get the total count of lines
|
|
int GetTotalLinesCount() { return TextLines.size(); };
|
|
//!Get the original full Text
|
|
const wchar_t * GetText();
|
|
//!Get the original full Text as wString
|
|
wString * GetwString() { return wText; };
|
|
//!Get the original Text as a UTF-8 text
|
|
std::string GetUTF8String() const;
|
|
//!Get a Textline
|
|
const wchar_t * GetTextLine(int ind);
|
|
//!Get the offset in the text of a drawn Line
|
|
int GetLineOffset(int ind);
|
|
//!Constantly called to draw the text
|
|
void Draw();
|
|
protected:
|
|
void CalcLineOffsets();
|
|
void FillRows();
|
|
|
|
wString * wText;
|
|
std::vector<TextLine> TextLines;
|
|
int curLineStart;
|
|
int FirstLineOffset;
|
|
bool filling;
|
|
};
|
|
|
|
#endif
|