mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-10-01 03:18:36 +02:00
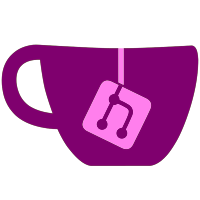
*Created a checkbox class with them *Changed the prompt window to a class *Changed cover download to a checkbox selection of what you want to download. Changed discart download option to only switch between what is prioritized (original/customs) *Fixed a little alignment issue of the progressbar *Reverted the change for check disc in drive. Some drives couldn't handle it in IOS58 mode :\. *Changed playcount from an 8 bit to a 32 bit counter (got 8bit by mistake) which limited it to 255 *a few little optimisations
64 lines
2.0 KiB
C++
64 lines
2.0 KiB
C++
/****************************************************************************
|
|
* Copyright (C) 2010
|
|
* by Dimok
|
|
*
|
|
* This software is provided 'as-is', without any express or implied
|
|
* warranty. In no event will the authors be held liable for any
|
|
* damages arising from the use of this software.
|
|
*
|
|
* Permission is granted to anyone to use this software for any
|
|
* purpose, including commercial applications, and to alter it and
|
|
* redistribute it freely, subject to the following restrictions:
|
|
*
|
|
* 1. The origin of this software must not be misrepresented; you
|
|
* must not claim that you wrote the original software. If you use
|
|
* this software in a product, an acknowledgment in the product
|
|
* documentation would be appreciated but is not required.
|
|
*
|
|
* 2. Altered source versions must be plainly marked as such, and
|
|
* must not be misrepresented as being the original software.
|
|
*
|
|
* 3. This notice may not be removed or altered from any source
|
|
* distribution.
|
|
***************************************************************************/
|
|
#include "ThreadedTask.hpp"
|
|
|
|
ThreadedTask * ThreadedTask::instance = NULL;
|
|
|
|
ThreadedTask::ThreadedTask()
|
|
: ExitRequested(false)
|
|
{
|
|
LWP_CreateThread (&Thread, ThreadCallback, this, NULL, 16384, 80);
|
|
}
|
|
|
|
ThreadedTask::~ThreadedTask()
|
|
{
|
|
ExitRequested = true;
|
|
Execute();
|
|
LWP_JoinThread(Thread, NULL);
|
|
}
|
|
|
|
void * ThreadedTask::ThreadCallback(void *arg)
|
|
{
|
|
ThreadedTask * myInstance = (ThreadedTask *) arg;
|
|
|
|
while(!myInstance->ExitRequested)
|
|
{
|
|
LWP_SuspendThread(myInstance->Thread);
|
|
|
|
while(!myInstance->CallbackList.empty())
|
|
{
|
|
if(myInstance->CallbackList[0].first)
|
|
myInstance->CallbackList[0].first->Execute(myInstance->ArgList[0]);
|
|
|
|
else if(myInstance->CallbackList[0].second)
|
|
myInstance->CallbackList[0].second(myInstance->ArgList[0]);
|
|
|
|
myInstance->CallbackList.erase(myInstance->CallbackList.begin());
|
|
myInstance->ArgList.erase(myInstance->ArgList.begin());
|
|
}
|
|
}
|
|
|
|
return NULL;
|
|
}
|