mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-06-27 21:46:05 +02:00
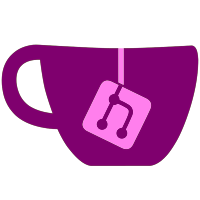
*Added sources of the custom libs to the branches *Fixed crash when switching from list layout to grid/carousel layout *Removed 1:1 copy option because its meaningless and almost the same as installing all partitions *Fixed install partition selection. This option needs a reset. Go to settings and reselect your option for this. *Fixed schinese and tchinese language modes (filename bugs. has to be schinese.lang and tchinese.lang like on SVN) *Fixed bug in sound buffer circle *Fixed incorrect behaviour of x-flip when selecting system like (thx Cyan for the patch) *Accept ios revision 65535 for Waninkokos IOSes (thx to PPSainity for pointing it out) *Merged the new theming style branch into trunk. Just as a reminder: ALL old themes will not work until the themers did port it to the new style! *Removed old theme style completely Theme example: The example file of the theme is the Default.them file. It can be found in the SVN trunk. Change in loading of themes: When selecting a theme now a list of all .them files in a folder is displayed. The image folder of that theme has to be in the same folder as the .them file. The image path is defined in the head of the .them file in the line with "Image-Folder: Example\n".
58 lines
1.7 KiB
C
58 lines
1.7 KiB
C
/***************************************************************************
|
|
* Copyright (C) 2010
|
|
* by dude, Dimok
|
|
*
|
|
* This software is provided 'as-is', without any express or implied
|
|
* warranty. In no event will the authors be held liable for any
|
|
* damages arising from the use of this software.
|
|
*
|
|
* Permission is granted to anyone to use this software for any
|
|
* purpose, including commercial applications, and to alter it and
|
|
* redistribute it freely, subject to the following restrictions:
|
|
*
|
|
* 1. The origin of this software must not be misrepresented; you
|
|
* must not claim that you wrote the original software. If you use
|
|
* this software in a product, an acknowledgment in the product
|
|
* documentation would be appreciated but is not required.
|
|
*
|
|
* 2. Altered source versions must be plainly marked as such, and
|
|
* must not be misrepresented as being the original software.
|
|
*
|
|
* 3. This notice may not be removed or altered from any source
|
|
* distribution.
|
|
*
|
|
* for WiiXplorer 2010
|
|
***************************************************************************/
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
|
|
//! No need for high security crap. It's a simple encrypter/decrypter
|
|
//! with a constant sid.
|
|
const char * sid = "USBLoaderGX";
|
|
|
|
void EncryptString(const char *src, char *dst)
|
|
{
|
|
unsigned int i;
|
|
char tmp[3];
|
|
dst[0] = 0;
|
|
|
|
for (i = 0; i < strlen(src); i++)
|
|
{
|
|
sprintf(tmp, "%02x", src[i] ^ sid[i%10]);
|
|
strcat(dst, tmp);
|
|
}
|
|
}
|
|
|
|
void DecryptString(const char *src, char *dst)
|
|
{
|
|
unsigned int i;
|
|
for (i = 0; i < strlen(src); i += 2)
|
|
{
|
|
char c = (src[i] >= 'a' ? (src[i] - 'a') + 10 : (src[i] - '0')) << 4;
|
|
c += (src[i+1] >= 'a' ? (src[i+1] - 'a') + 10 : (src[i+1] - '0'));
|
|
dst[i>>1] = c ^ sid[(i>>1)%10];
|
|
}
|
|
dst[strlen(src)>>1] = 0;
|
|
}
|
|
|