mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-04 00:46:10 +02:00
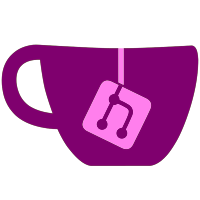
*Fixed reset of the loader when loading game with IOS reload and disabled WiiTDB titles (Issue 1874) *Added new 'Inherit' or 'Use global' setting for game settings. If that option is set than the global setting is used for that option. (Issue 1842) *Fixed timeout timer on startup to count correctly. (Issue 1907) *Added two new video modes to force progressive video mode, 'FORCE PAL480p' and 'FORCE NTSC480p' (basically the same but oh well) (Issue 1902) *Added the new 'Return to' procedure for the d2x v4 IOS for Test purpose (didn't test it, need feedback on this one). The old method is used if this procedure fails. Please test it on problematic games (e.g. PoP). (Issue 1892)
75 lines
2.1 KiB
C++
75 lines
2.1 KiB
C++
#ifndef _GAME_SETTINGS_H_
|
|
#define _GAME_SETTINGS_H_
|
|
|
|
#include <string>
|
|
#include <stdio.h>
|
|
#include <gctypes.h>
|
|
#include <vector>
|
|
#include "usbloader/disc.h"
|
|
|
|
typedef struct _GameCFG
|
|
{
|
|
char id[7];
|
|
short video;
|
|
short language;
|
|
short ocarina;
|
|
short vipatch;
|
|
short ios;
|
|
short parentalcontrol;
|
|
short errorfix002;
|
|
short iosreloadblock;
|
|
short loadalternatedol;
|
|
u32 alternatedolstart;
|
|
short patchcountrystrings;
|
|
char alternatedolname[40];
|
|
short returnTo;
|
|
short Locked;
|
|
} GameCFG;
|
|
|
|
class CGameSettings
|
|
{
|
|
public:
|
|
//!Constructor
|
|
CGameSettings();
|
|
//!Destructor
|
|
~CGameSettings();
|
|
//!Load
|
|
bool Load(const char * path);
|
|
//!Save
|
|
bool Save();
|
|
//!AddGame
|
|
bool AddGame(const GameCFG & NewGame);
|
|
//!Reset
|
|
bool RemoveAll();
|
|
//!Overload Reset for one Game
|
|
bool Remove(const char * id);
|
|
bool Remove(const u8 * id) { return Remove((const char *) id); };
|
|
bool Remove(const struct discHdr * game) { if(!game) return false; else return Remove(game->id); };
|
|
//!Get GameCFG
|
|
GameCFG * GetGameCFG(const char * id);
|
|
//!Overload
|
|
GameCFG * GetGameCFG(const u8 * id) { return GetGameCFG((const char *) id); };
|
|
//!Overload
|
|
GameCFG * GetGameCFG(const struct discHdr * game) { if(!game) return NULL; else return GetGameCFG(game->id); };
|
|
//!Quick settings to PEGI conversion
|
|
static int GetPartenalPEGI(int parentalsetting);
|
|
//!Get the default configuration block
|
|
GameCFG * GetDefault();
|
|
protected:
|
|
bool ReadGameID(const char * src, char * GameID, int size);
|
|
bool SetSetting(GameCFG & game, char *name, char *value);
|
|
bool ValidVersion(FILE * file);
|
|
//!Find the config file in the default paths
|
|
bool FindConfig();
|
|
|
|
void ParseLine(char *line);
|
|
void TrimLine(char *dest, const char *src, int size);
|
|
std::string ConfigPath;
|
|
std::vector<GameCFG> GameList;
|
|
GameCFG DefaultConfig;
|
|
};
|
|
|
|
extern CGameSettings GameSettings;
|
|
|
|
#endif
|