mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-06-29 22:46:07 +02:00
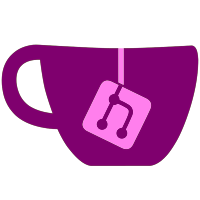
the "GameCube Source" setting has USB priority. * Fixed Playlog writing when using Hermes cIOS v4 (untested) (Requires AHB access). * Fixed EmuNAND when using cIOS revision 65535 (issue 2225) * Added Nintendont support: 1. Select Nintendont's boot.dol folder in userpath settings. 2. Set the "GameCube Mode" setting to Nintendont. 3. Nintendont share some of DIOS MIOS (Lite) settings. * Added sections in the Loader settings (Wii/gamecube/Devolution/DIOS MIOS/Nintendont). * Updated the GameCube game settings to display only the selected GameCube mode related settings. * Updated some menus to support more controller's input: - Prevent GC/CC X and Y buttons to change row number in Wall layout (use d-pad up/down only) - Added GC/CC support to carousel's arrow button - Added GC/CC support to Wall/Carousel continuous scroll (+/- on CC, L/R on GC) - Added GC support L/R and Start buttons in the settings/homebrew browser. - Added D-pad support in listing windows if not pointing the screen. The cursor now moves with the selection (not very good with high Overscan value) (issue 2093) * Changed the StartupProcess to speed up launch time by using AHB access to read config files. IOS argument in meta.xml has priority over AHB detection. * Added IOS58 + AHB support for launching the loader without cIOS (Wii games and EmuNAND still require cIOS). * Added a Loader's IOS setting (now Loader and Games use two separate settings: loader can use 58 and games 249). * Added LibruntimeIOSPatch to patch IOS58 and Hermes v4 to get ISFS access and enable Banner mode, Channel's title and System font with these IOSes (Requires AHB access) * Added a delete prompt if downloaded cheat file is empty. * Force all launched homebrew to reload to IOS58 if available. * Changed Gecko.c to send logs to wifigecko too. * Changed wifigecko IP to send logs to all IP 192.168.0.x * Updated French translation.
78 lines
1.4 KiB
C
78 lines
1.4 KiB
C
#ifndef _DISC_H_
|
|
#define _DISC_H_
|
|
|
|
#include <gccore.h> /* for define ATTRIBUTE_PACKED */
|
|
|
|
#ifdef __cplusplus
|
|
extern "C"
|
|
{
|
|
#endif
|
|
|
|
/* Disc header structure */
|
|
struct discHdr
|
|
{
|
|
/* Game ID */
|
|
u8 id[6];
|
|
|
|
/* Game Disc number */
|
|
u8 disc_no;
|
|
|
|
/* Game version */
|
|
u8 disc_ver;
|
|
|
|
/* Audio streaming */
|
|
u8 streaming;
|
|
u8 bufsize;
|
|
|
|
/* Padding */
|
|
u8 is_ciso;
|
|
|
|
/* Unused, on channel list mode this is the full 64 bit tid */
|
|
u64 tid;
|
|
|
|
/* Unused, using in loader internally to detect title type */
|
|
u8 type;
|
|
|
|
/* rest of unused */
|
|
u8 unused[4];
|
|
|
|
/* Magic word */
|
|
u32 magic;
|
|
|
|
/* Padding */
|
|
u32 gc_magic;
|
|
|
|
/* Game title */
|
|
char title[64];
|
|
|
|
/* Encryption/Hashing */
|
|
u8 encryption;
|
|
u8 h3_verify;
|
|
|
|
/* Padding */
|
|
u8 unused3[30];
|
|
} ATTRIBUTE_PACKED;
|
|
|
|
/* Prototypes */
|
|
s32 Disc_Init(void);
|
|
s32 Disc_Open(void);
|
|
s32 Disc_Wait(void);
|
|
void Disc_SetLowMem(void);
|
|
s32 Disc_SetUSB(const u8 *);
|
|
s32 Disc_ReadHeader(void *);
|
|
s32 Disc_IsWii(void);
|
|
s32 Disc_FindPartition(u64 *outbuf);
|
|
s32 Disc_Mount(struct discHdr *header);
|
|
void PatchCountryStrings(void *Address, int Size);
|
|
void Disc_SelectVMode(u8 videoselected, bool devolution, u32 *dml_VideoMode, u32 *nin_VideoMode);
|
|
void Disc_SetVMode(void);
|
|
s32 Disc_JumpToEntrypoint(s32 hooktype, u32 dolparameter);
|
|
|
|
extern GXRModeObj *rmode;
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif
|