mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-01 15:36:08 +02:00
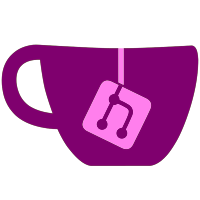
*added channel hooking (real and emu). *proper catch of none existing cheats on server if file is not found *added back channel nand emulation mode and per game nand emu settings for channels (emulation mode can't be set to OFF for emu channels) *improved handle of cached titles. (on first boot of this revision the cache title file will be renewed) *settings title, keyboard text and key color can be changed by themes now *added optional background image for list/carousel/grid layouts. The files do not actually exist in the loader. They are loaded if they exists otherwise the default background image is used.See here for filenames: http://code.google.com/p/usbloader-gui/source/browse/trunk/source/themes/filelist.h?r=1148#776 *add flush of homebrew memory before launching app_booter *change: if titles from wiitdb is not enabled the title will be read from game disc header on /title [ID6]/[ID6].wbfs (iso/ciso) pattern. Title caching can still be used and is recommended in that case. *added keep of AHBPROT flag on IOS Reload. Homebrews booted now have AHBPROT. (thanks davebaol) *fixed loading ocarina files from SD when doing nand emulation from SD *fix port libs includes reference in Makefile
56 lines
1.5 KiB
C++
56 lines
1.5 KiB
C++
#ifndef GAMETDB_TITLES_H_
|
|
#define GAMETDB_TITLES_H_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <gctypes.h>
|
|
#include "usbloader/disc.h"
|
|
|
|
typedef struct _GameTitle
|
|
{
|
|
char GameID[7];
|
|
std::string Title;
|
|
int ParentalRating;
|
|
int PlayersCount;
|
|
char FromWiiTDB;
|
|
|
|
} GameTitle;
|
|
|
|
class CGameTitles
|
|
{
|
|
public:
|
|
//! Set a game title from GameTDB
|
|
void SetGameTitle(const char * id, const char * title);
|
|
//! Overload
|
|
void SetGameTitle(const u8 * id, const char * title) { SetGameTitle((const char *) id, title); };
|
|
|
|
//! Get a game title
|
|
const char * GetTitle(const char * id) const;
|
|
//! Overload
|
|
const char * GetTitle(const u8 * id) const { return GetTitle((const char *) id); };
|
|
//! Overload
|
|
const char * GetTitle(const struct discHdr *header) const;
|
|
|
|
//! Get game parental rating
|
|
int GetParentalRating(const char * id) const;
|
|
//! Get possible number of players for this game
|
|
int GetPlayersCount(const char * id) const;
|
|
//! Load Game Titles from GameTDB
|
|
void LoadTitlesFromGameTDB(const char * path, bool removeUnused = true);
|
|
//! Set default game titles
|
|
void SetDefault();
|
|
//! Free memory and remove all titles - Same as SetDefault()
|
|
void Clear() { SetDefault(); }
|
|
//! Cache titles functions
|
|
u32 ReadCachedTitles(const char * path);
|
|
void WriteCachedTitles(const char * path);
|
|
protected:
|
|
void GetMissingTitles(std::vector<std::string> &MissingTitles, bool removeUnused);
|
|
|
|
std::vector<GameTitle> TitleList;
|
|
};
|
|
|
|
extern CGameTitles GameTitles;
|
|
|
|
#endif
|