mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-06-27 05:26:06 +02:00
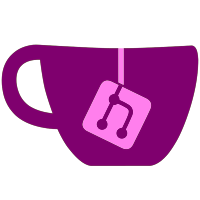
NOTE: The images used right now for the theme loader are made out of our old images and if someone can make some new ones go ahead. The themes are downloaded to the path you can set up. The downloaded preview images are cached at "<set up theme downloadpath>/tmp/". The widescreenfix isn't implemented there yet because images will probably be changed. *Started 3rd SettingsPage *Added JPEG Loading GuiImageData function and 4x4RGBA converter by r-win from WiiXplorer *Added ZipFile Class from WiiXplorer *Added StringToMD5 and MD5ToString to MD5 functions and made it undependable of platform
141 lines
3.7 KiB
C++
141 lines
3.7 KiB
C++
/****************************************************************************
|
|
* Copyright (C) 2009
|
|
* by Dimok
|
|
*
|
|
* This software is provided 'as-is', without any express or implied
|
|
* warranty. In no event will the authors be held liable for any
|
|
* damages arising from the use of this software.
|
|
*
|
|
* Permission is granted to anyone to use this software for any
|
|
* purpose, including commercial applications, and to alter it and
|
|
* redistribute it freely, subject to the following restrictions:
|
|
*
|
|
* 1. The origin of this software must not be misrepresented; you
|
|
* must not claim that you wrote the original software. If you use
|
|
* this software in a product, an acknowledgment in the product
|
|
* documentation would be appreciated but is not required.
|
|
*
|
|
* 2. Altered source versions must be plainly marked as such, and
|
|
* must not be misrepresented as being the original software.
|
|
*
|
|
* 3. This notice may not be removed or altered from any source
|
|
* distribution.
|
|
*
|
|
* ZipFile.cpp
|
|
*
|
|
* ZipFile Class
|
|
* for Wii-FileXplorer 2009
|
|
*
|
|
* STILL UNCOMPLETE AND UNDER CONSTRUCTION
|
|
***************************************************************************/
|
|
#include <ogcsys.h>
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <malloc.h>
|
|
|
|
#include "prompts/ProgressWindow.h"
|
|
#include "listfiles.h"
|
|
#include "ZipFile.h"
|
|
#include "language/gettext.h"
|
|
|
|
ZipFile::ZipFile(const char *filepath)
|
|
{
|
|
File = unzOpen(filepath);
|
|
if(File)
|
|
this->LoadList();
|
|
}
|
|
|
|
ZipFile::~ZipFile()
|
|
{
|
|
unzClose(File);
|
|
}
|
|
|
|
bool ZipFile::LoadList()
|
|
{
|
|
return true;
|
|
}
|
|
|
|
bool ZipFile::ExtractAll(const char *dest)
|
|
{
|
|
if(!File)
|
|
return false;
|
|
|
|
bool Stop = false;
|
|
|
|
u32 blocksize = 1024*50;
|
|
void *buffer = malloc(blocksize);
|
|
|
|
if(!buffer)
|
|
return false;
|
|
|
|
char writepath[MAXPATHLEN];
|
|
char filename[MAXPATHLEN];
|
|
memset(filename, 0, sizeof(filename));
|
|
|
|
int ret = unzGoToFirstFile(File);
|
|
if(ret != UNZ_OK)
|
|
Stop = true;
|
|
|
|
while(!Stop)
|
|
{
|
|
if(unzGetCurrentFileInfo(File, &cur_file_info, filename, sizeof(filename), NULL, NULL, NULL, NULL) != UNZ_OK)
|
|
Stop = true;
|
|
|
|
if(!Stop && filename[strlen(filename)-1] != '/')
|
|
{
|
|
u32 uncompressed_size = cur_file_info.uncompressed_size;
|
|
|
|
u32 done = 0;
|
|
char *pointer = NULL;
|
|
|
|
ret = unzOpenCurrentFile(File);
|
|
|
|
snprintf(writepath, sizeof(writepath), "%s/%s", dest, filename);
|
|
|
|
pointer = strrchr(writepath, '/');
|
|
int position = pointer-writepath+2;
|
|
|
|
char temppath[strlen(writepath)];
|
|
snprintf(temppath, position, "%s", writepath);
|
|
|
|
subfoldercreate(temppath);
|
|
|
|
if(ret == UNZ_OK)
|
|
{
|
|
FILE *pfile = fopen(writepath, "wb");
|
|
|
|
do
|
|
{
|
|
ShowProgress(tr("Extracting files..."), 0, pointer+1, done, uncompressed_size);
|
|
|
|
if(uncompressed_size - done < blocksize)
|
|
blocksize = uncompressed_size - done;
|
|
|
|
ret = unzReadCurrentFile(File, buffer, blocksize);
|
|
|
|
if(ret == 0)
|
|
break;
|
|
|
|
fwrite(buffer, 1, blocksize, pfile);
|
|
|
|
done += ret;
|
|
|
|
} while(done < uncompressed_size);
|
|
|
|
fclose(pfile);
|
|
unzCloseCurrentFile(File);
|
|
}
|
|
}
|
|
if(unzGoToNextFile(File) != UNZ_OK)
|
|
Stop = true;
|
|
}
|
|
|
|
free(buffer);
|
|
buffer = NULL;
|
|
|
|
ProgressStop();
|
|
|
|
return true;
|
|
}
|