mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-06 01:41:49 +02:00
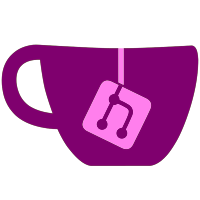
*Reworked almost everything about alternate DOL loading (I am wondering how loading from SD/USB even worked) *Added new Option "Default" for alternate DOL which is now the default settings. This setting will select the alternate DOL automatically for games like Red Steel 1 because this alt. DOL is always needed to play the game. On games where the alt dol option is not needed the option "Default" = "OFF". This is just an attempt to make this option more automatic. Games like Metroid Prime (the only game right now) will have a prompt on game start where you choose between 1, 2 or 3 on this option because this game always requires either of these. *Small source cleanups/fixes
75 lines
2.1 KiB
C++
75 lines
2.1 KiB
C++
#ifndef _GAME_SETTINGS_H_
|
|
#define _GAME_SETTINGS_H_
|
|
|
|
#include <string>
|
|
#include <stdio.h>
|
|
#include <gctypes.h>
|
|
#include <vector>
|
|
#include "usbloader/disc.h"
|
|
|
|
typedef struct _GameCFG
|
|
{
|
|
char id[7];
|
|
u8 video;
|
|
u8 language;
|
|
u8 ocarina;
|
|
u8 vipatch;
|
|
u8 ios;
|
|
u8 parentalcontrol;
|
|
u8 errorfix002;
|
|
u8 iosreloadblock;
|
|
u8 loadalternatedol;
|
|
u32 alternatedolstart;
|
|
u8 patchcountrystrings;
|
|
char alternatedolname[40];
|
|
u8 returnTo;
|
|
u8 Locked;
|
|
} GameCFG;
|
|
|
|
class CGameSettings
|
|
{
|
|
public:
|
|
//!Constructor
|
|
CGameSettings();
|
|
//!Destructor
|
|
~CGameSettings();
|
|
//!Load
|
|
bool Load(const char * path);
|
|
//!Save
|
|
bool Save();
|
|
//!AddGame
|
|
bool AddGame(const GameCFG & NewGame);
|
|
//!Reset
|
|
bool RemoveAll();
|
|
//!Overload Reset for one Game
|
|
bool Remove(const char * id);
|
|
bool Remove(const u8 * id) { return Remove((const char *) id); };
|
|
bool Remove(const struct discHdr * game) { if(!game) return false; else return Remove(game->id); };
|
|
//!Get GameCFG
|
|
GameCFG * GetGameCFG(const char * id);
|
|
//!Overload
|
|
GameCFG * GetGameCFG(const u8 * id) { return GetGameCFG((const char *) id); };
|
|
//!Overload
|
|
GameCFG * GetGameCFG(const struct discHdr * game) { if(!game) return NULL; else return GetGameCFG(game->id); };
|
|
//!Quick settings to PEGI conversion
|
|
static int GetPartenalPEGI(int parentalsetting);
|
|
//!Get the default configuration block
|
|
GameCFG * GetDefault();
|
|
protected:
|
|
bool ReadGameID(const char * src, char * GameID, int size);
|
|
bool SetSetting(GameCFG & game, char *name, char *value);
|
|
bool ValidVersion(FILE * file);
|
|
//!Find the config file in the default paths
|
|
bool FindConfig();
|
|
|
|
void ParseLine(char *line);
|
|
void TrimLine(char *dest, const char *src, int size);
|
|
std::string ConfigPath;
|
|
std::vector<GameCFG> GameList;
|
|
GameCFG DefaultConfig;
|
|
};
|
|
|
|
extern CGameSettings GameSettings;
|
|
|
|
#endif
|