mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-11-16 00:15:08 +01:00
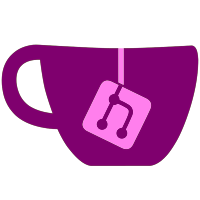
*Added sources of the custom libs to the branches *Fixed crash when switching from list layout to grid/carousel layout *Removed 1:1 copy option because its meaningless and almost the same as installing all partitions *Fixed install partition selection. This option needs a reset. Go to settings and reselect your option for this. *Fixed schinese and tchinese language modes (filename bugs. has to be schinese.lang and tchinese.lang like on SVN) *Fixed bug in sound buffer circle *Fixed incorrect behaviour of x-flip when selecting system like (thx Cyan for the patch) *Accept ios revision 65535 for Waninkokos IOSes (thx to PPSainity for pointing it out) *Merged the new theming style branch into trunk. Just as a reminder: ALL old themes will not work until the themers did port it to the new style! *Removed old theme style completely Theme example: The example file of the theme is the Default.them file. It can be found in the SVN trunk. Change in loading of themes: When selecting a theme now a list of all .them files in a folder is displayed. The image folder of that theme has to be in the same folder as the .them file. The image path is defined in the head of the .them file in the line with "Image-Folder: Example\n".
328 lines
6.4 KiB
C
328 lines
6.4 KiB
C
/*
|
|
* badblocks.c --- routines to manipulate the bad block structure
|
|
*
|
|
* Copyright (C) 1994, 1995, 1996 Theodore Ts'o.
|
|
*
|
|
* %Begin-Header%
|
|
* This file may be redistributed under the terms of the GNU Library
|
|
* General Public License, version 2.
|
|
* %End-Header%
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#if HAVE_UNISTD_H
|
|
#include <unistd.h>
|
|
#endif
|
|
#include <fcntl.h>
|
|
#include <time.h>
|
|
#if HAVE_SYS_STAT_H
|
|
#include <sys/stat.h>
|
|
#endif
|
|
#if HAVE_SYS_TYPES_H
|
|
#include <sys/types.h>
|
|
#endif
|
|
|
|
#include "ext2_fs.h"
|
|
#include "ext2fsP.h"
|
|
|
|
/*
|
|
* Helper function for making a badblocks list
|
|
*/
|
|
static errcode_t make_u32_list(int size, int num, __u32 *list,
|
|
ext2_u32_list *ret)
|
|
{
|
|
ext2_u32_list bb;
|
|
errcode_t retval;
|
|
|
|
retval = ext2fs_get_mem(sizeof(struct ext2_struct_u32_list), &bb);
|
|
if (retval)
|
|
return retval;
|
|
memset(bb, 0, sizeof(struct ext2_struct_u32_list));
|
|
bb->magic = EXT2_ET_MAGIC_BADBLOCKS_LIST;
|
|
bb->size = size ? size : 10;
|
|
bb->num = num;
|
|
retval = ext2fs_get_array(bb->size, sizeof(blk_t), &bb->list);
|
|
if (retval) {
|
|
ext2fs_free_mem(&bb);
|
|
return retval;
|
|
}
|
|
if (list)
|
|
memcpy(bb->list, list, bb->size * sizeof(blk_t));
|
|
else
|
|
memset(bb->list, 0, bb->size * sizeof(blk_t));
|
|
*ret = bb;
|
|
return 0;
|
|
}
|
|
|
|
|
|
/*
|
|
* This procedure creates an empty u32 list.
|
|
*/
|
|
errcode_t ext2fs_u32_list_create(ext2_u32_list *ret, int size)
|
|
{
|
|
return make_u32_list(size, 0, 0, ret);
|
|
}
|
|
|
|
/*
|
|
* This procedure creates an empty badblocks list.
|
|
*/
|
|
errcode_t ext2fs_badblocks_list_create(ext2_badblocks_list *ret, int size)
|
|
{
|
|
return make_u32_list(size, 0, 0, (ext2_badblocks_list *) ret);
|
|
}
|
|
|
|
|
|
/*
|
|
* This procedure copies a badblocks list
|
|
*/
|
|
errcode_t ext2fs_u32_copy(ext2_u32_list src, ext2_u32_list *dest)
|
|
{
|
|
errcode_t retval;
|
|
|
|
retval = make_u32_list(src->size, src->num, src->list, dest);
|
|
if (retval)
|
|
return retval;
|
|
(*dest)->badblocks_flags = src->badblocks_flags;
|
|
return 0;
|
|
}
|
|
|
|
errcode_t ext2fs_badblocks_copy(ext2_badblocks_list src,
|
|
ext2_badblocks_list *dest)
|
|
{
|
|
return ext2fs_u32_copy((ext2_u32_list) src,
|
|
(ext2_u32_list *) dest);
|
|
}
|
|
|
|
/*
|
|
* This procedure frees a badblocks list.
|
|
*
|
|
* (note: moved to closefs.c)
|
|
*/
|
|
|
|
|
|
/*
|
|
* This procedure adds a block to a badblocks list.
|
|
*/
|
|
errcode_t ext2fs_u32_list_add(ext2_u32_list bb, __u32 blk)
|
|
{
|
|
errcode_t retval;
|
|
int i, j;
|
|
unsigned long old_size;
|
|
|
|
EXT2_CHECK_MAGIC(bb, EXT2_ET_MAGIC_BADBLOCKS_LIST);
|
|
|
|
if (bb->num >= bb->size) {
|
|
old_size = bb->size * sizeof(__u32);
|
|
bb->size += 100;
|
|
retval = ext2fs_resize_mem(old_size, bb->size * sizeof(__u32),
|
|
&bb->list);
|
|
if (retval) {
|
|
bb->size -= 100;
|
|
return retval;
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Add special case code for appending to the end of the list
|
|
*/
|
|
i = bb->num-1;
|
|
if ((bb->num != 0) && (bb->list[i] == blk))
|
|
return 0;
|
|
if ((bb->num == 0) || (bb->list[i] < blk)) {
|
|
bb->list[bb->num++] = blk;
|
|
return 0;
|
|
}
|
|
|
|
j = bb->num;
|
|
for (i=0; i < bb->num; i++) {
|
|
if (bb->list[i] == blk)
|
|
return 0;
|
|
if (bb->list[i] > blk) {
|
|
j = i;
|
|
break;
|
|
}
|
|
}
|
|
for (i=bb->num; i > j; i--)
|
|
bb->list[i] = bb->list[i-1];
|
|
bb->list[j] = blk;
|
|
bb->num++;
|
|
return 0;
|
|
}
|
|
|
|
errcode_t ext2fs_badblocks_list_add(ext2_badblocks_list bb, blk_t blk)
|
|
{
|
|
return ext2fs_u32_list_add((ext2_u32_list) bb, (__u32) blk);
|
|
}
|
|
|
|
/*
|
|
* This procedure finds a particular block is on a badblocks
|
|
* list.
|
|
*/
|
|
int ext2fs_u32_list_find(ext2_u32_list bb, __u32 blk)
|
|
{
|
|
int low, high, mid;
|
|
|
|
if (bb->magic != EXT2_ET_MAGIC_BADBLOCKS_LIST)
|
|
return -1;
|
|
|
|
if (bb->num == 0)
|
|
return -1;
|
|
|
|
low = 0;
|
|
high = bb->num-1;
|
|
if (blk == bb->list[low])
|
|
return low;
|
|
if (blk == bb->list[high])
|
|
return high;
|
|
|
|
while (low < high) {
|
|
mid = (low+high)/2;
|
|
if (mid == low || mid == high)
|
|
break;
|
|
if (blk == bb->list[mid])
|
|
return mid;
|
|
if (blk < bb->list[mid])
|
|
high = mid;
|
|
else
|
|
low = mid;
|
|
}
|
|
return -1;
|
|
}
|
|
|
|
/*
|
|
* This procedure tests to see if a particular block is on a badblocks
|
|
* list.
|
|
*/
|
|
int ext2fs_u32_list_test(ext2_u32_list bb, __u32 blk)
|
|
{
|
|
if (ext2fs_u32_list_find(bb, blk) < 0)
|
|
return 0;
|
|
else
|
|
return 1;
|
|
}
|
|
|
|
int ext2fs_badblocks_list_test(ext2_badblocks_list bb, blk_t blk)
|
|
{
|
|
return ext2fs_u32_list_test((ext2_u32_list) bb, (__u32) blk);
|
|
}
|
|
|
|
|
|
/*
|
|
* Remove a block from the badblock list
|
|
*/
|
|
int ext2fs_u32_list_del(ext2_u32_list bb, __u32 blk)
|
|
{
|
|
int remloc, i;
|
|
|
|
if (bb->num == 0)
|
|
return -1;
|
|
|
|
remloc = ext2fs_u32_list_find(bb, blk);
|
|
if (remloc < 0)
|
|
return -1;
|
|
|
|
for (i = remloc ; i < bb->num-1; i++)
|
|
bb->list[i] = bb->list[i+1];
|
|
bb->num--;
|
|
return 0;
|
|
}
|
|
|
|
void ext2fs_badblocks_list_del(ext2_u32_list bb, __u32 blk)
|
|
{
|
|
ext2fs_u32_list_del(bb, blk);
|
|
}
|
|
|
|
errcode_t ext2fs_u32_list_iterate_begin(ext2_u32_list bb,
|
|
ext2_u32_iterate *ret)
|
|
{
|
|
ext2_u32_iterate iter;
|
|
errcode_t retval;
|
|
|
|
EXT2_CHECK_MAGIC(bb, EXT2_ET_MAGIC_BADBLOCKS_LIST);
|
|
|
|
retval = ext2fs_get_mem(sizeof(struct ext2_struct_u32_iterate), &iter);
|
|
if (retval)
|
|
return retval;
|
|
|
|
iter->magic = EXT2_ET_MAGIC_BADBLOCKS_ITERATE;
|
|
iter->bb = bb;
|
|
iter->ptr = 0;
|
|
*ret = iter;
|
|
return 0;
|
|
}
|
|
|
|
errcode_t ext2fs_badblocks_list_iterate_begin(ext2_badblocks_list bb,
|
|
ext2_badblocks_iterate *ret)
|
|
{
|
|
return ext2fs_u32_list_iterate_begin((ext2_u32_list) bb,
|
|
(ext2_u32_iterate *) ret);
|
|
}
|
|
|
|
|
|
int ext2fs_u32_list_iterate(ext2_u32_iterate iter, __u32 *blk)
|
|
{
|
|
ext2_u32_list bb;
|
|
|
|
if (iter->magic != EXT2_ET_MAGIC_BADBLOCKS_ITERATE)
|
|
return 0;
|
|
|
|
bb = iter->bb;
|
|
|
|
if (bb->magic != EXT2_ET_MAGIC_BADBLOCKS_LIST)
|
|
return 0;
|
|
|
|
if (iter->ptr < bb->num) {
|
|
*blk = bb->list[iter->ptr++];
|
|
return 1;
|
|
}
|
|
*blk = 0;
|
|
return 0;
|
|
}
|
|
|
|
int ext2fs_badblocks_list_iterate(ext2_badblocks_iterate iter, blk_t *blk)
|
|
{
|
|
return ext2fs_u32_list_iterate((ext2_u32_iterate) iter,
|
|
(__u32 *) blk);
|
|
}
|
|
|
|
|
|
void ext2fs_u32_list_iterate_end(ext2_u32_iterate iter)
|
|
{
|
|
if (!iter || (iter->magic != EXT2_ET_MAGIC_BADBLOCKS_ITERATE))
|
|
return;
|
|
|
|
iter->bb = 0;
|
|
ext2fs_free_mem(&iter);
|
|
}
|
|
|
|
void ext2fs_badblocks_list_iterate_end(ext2_badblocks_iterate iter)
|
|
{
|
|
ext2fs_u32_list_iterate_end((ext2_u32_iterate) iter);
|
|
}
|
|
|
|
|
|
int ext2fs_u32_list_equal(ext2_u32_list bb1, ext2_u32_list bb2)
|
|
{
|
|
EXT2_CHECK_MAGIC(bb1, EXT2_ET_MAGIC_BADBLOCKS_LIST);
|
|
EXT2_CHECK_MAGIC(bb2, EXT2_ET_MAGIC_BADBLOCKS_LIST);
|
|
|
|
if (bb1->num != bb2->num)
|
|
return 0;
|
|
|
|
if (memcmp(bb1->list, bb2->list, bb1->num * sizeof(blk_t)) != 0)
|
|
return 0;
|
|
return 1;
|
|
}
|
|
|
|
int ext2fs_badblocks_equal(ext2_badblocks_list bb1, ext2_badblocks_list bb2)
|
|
{
|
|
return ext2fs_u32_list_equal((ext2_u32_list) bb1,
|
|
(ext2_u32_list) bb2);
|
|
}
|
|
|
|
int ext2fs_u32_list_count(ext2_u32_list bb)
|
|
{
|
|
return bb->num;
|
|
}
|