mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-09-30 19:08:36 +02:00
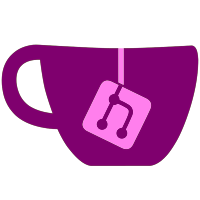
* Added auto-detection and loading multi-Disc games with both Devolution and DIOS MIOS (Lite). The Disc 2 must be named disc2.iso and placed in the same folder than game.iso * DML: Prevent temporary ocarina .gct file copy if the game is launched from disc Note: If you use "GameCube compress" option (Extracted game format): - If one of the disc is already extracted, it will not allows you to dump another disc (as it's now using the same folder, it would overwrite files from the other disc) - If Disc 1 doesn't exist, it will tell you that disc 2 needs to be in ISO format, but will asks if you really want to install the game in extracted format.
76 lines
1.3 KiB
C
76 lines
1.3 KiB
C
#ifndef _DISC_H_
|
|
#define _DISC_H_
|
|
|
|
#include <gccore.h> /* for define ATTRIBUTE_PACKED */
|
|
|
|
#ifdef __cplusplus
|
|
extern "C"
|
|
{
|
|
#endif
|
|
|
|
/* Disc header structure */
|
|
struct discHdr
|
|
{
|
|
/* Game ID */
|
|
u8 id[6];
|
|
|
|
/* Game Disc number */
|
|
u8 disc_no;
|
|
|
|
/* Game version */
|
|
u8 disc_ver;
|
|
|
|
/* Audio streaming */
|
|
u8 streaming;
|
|
u8 bufsize;
|
|
|
|
/* Padding */
|
|
u8 is_ciso;
|
|
|
|
/* Unused, on channel list mode this is the full 64 bit tid */
|
|
u64 tid;
|
|
|
|
/* Unused, using in loader internally to detect title type */
|
|
u8 type;
|
|
|
|
/* rest of unused */
|
|
u8 unused[4];
|
|
|
|
/* Magic word */
|
|
u32 magic;
|
|
|
|
/* Padding */
|
|
u32 gc_magic;
|
|
|
|
/* Game title */
|
|
char title[64];
|
|
|
|
/* Encryption/Hashing */
|
|
u8 encryption;
|
|
u8 h3_verify;
|
|
|
|
/* Padding */
|
|
u8 unused3[30];
|
|
} ATTRIBUTE_PACKED;
|
|
|
|
/* Prototypes */
|
|
s32 Disc_Init(void);
|
|
s32 Disc_Open(void);
|
|
s32 Disc_Wait(void);
|
|
void Disc_SetLowMem(void);
|
|
s32 Disc_SetUSB(const u8 *);
|
|
s32 Disc_ReadHeader(void *);
|
|
s32 Disc_IsWii(void);
|
|
s32 Disc_FindPartition(u64 *outbuf);
|
|
s32 Disc_Mount(struct discHdr *header);
|
|
void PatchCountryStrings(void *Address, int Size);
|
|
void Disc_SelectVMode(u8 videoselected, bool devolution, u32 *dml_VideoMode);
|
|
void Disc_SetVMode(void);
|
|
s32 Disc_JumpToEntrypoint(s32 hooktype, u32 dolparameter);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif
|