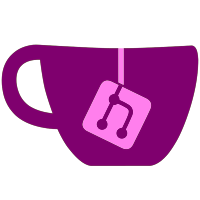
All help messages and many other things moved from markdown to html. Eventually, I'd like only things made from user input to use markdown. cats.lua, rmspic.lua, and dilbert.lua moved to sendPhoto with URL. xkcd.lua and apod.lua not moved to retain formatting. Probably a lot of other stuff that I forget about. I should commit more often.
71 lines
2.4 KiB
Lua
71 lines
2.4 KiB
Lua
local channel = {}
|
|
|
|
local bindings = require('otouto.bindings')
|
|
local utilities = require('otouto.utilities')
|
|
|
|
function channel:init(config)
|
|
channel.triggers = utilities.triggers(self.info.username, config.cmd_pat):t('ch', true).table
|
|
channel.command = 'ch <channel> \\n <message>'
|
|
channel.doc = config.cmd_pat .. [[ch <channel>
|
|
<message>
|
|
|
|
Sends a message to a channel. Channel may be specified via ID or username. Messages are markdown-enabled. Users may only send messages to channels for which they are the owner or an administrator.
|
|
|
|
The following markdown syntax is supported:
|
|
*bold text*
|
|
_italic text_
|
|
[text](URL)
|
|
`inline fixed-width code`
|
|
```pre-formatted fixed-width code block```]]
|
|
end
|
|
|
|
function channel:action(msg, config)
|
|
local input = utilities.input(msg.text)
|
|
if not input then
|
|
utilities.send_reply(msg, channel.doc, 'html')
|
|
return
|
|
end
|
|
|
|
local chat_id = utilities.get_word(input, 1)
|
|
local chat, t = bindings.getChat{chat_id = chat_id}
|
|
if not chat then
|
|
utilities.send_reply(msg, 'Sorry, I was unable to retrieve information for that channel.\n`' .. t.description .. '`', true)
|
|
return
|
|
elseif chat.result.type ~= 'channel' then
|
|
utilities.send_reply(msg, 'Sorry, that group does not appear to be a channel.')
|
|
return
|
|
end
|
|
|
|
local admin_list, t = bindings.getChatAdministrators{ chat_id = chat_id }
|
|
if not admin_list then
|
|
utilities.send_reply(msg, 'Sorry, I was unable to retrieve a list of administrators for that channel.\n`' .. t.description .. '`', true)
|
|
return
|
|
end
|
|
|
|
local is_admin = false
|
|
for _, admin in ipairs(admin_list.result) do
|
|
if admin.user.id == msg.from.id then
|
|
is_admin = true
|
|
end
|
|
end
|
|
if not is_admin then
|
|
utilities.send_reply(msg, 'Sorry, you do not appear to be an administrator for that channel.')
|
|
return
|
|
end
|
|
|
|
local text = input:match('\n(.+)')
|
|
if not text then
|
|
utilities.send_reply(msg, 'Please enter a message to be sent on a new line. Markdown is supported.')
|
|
return
|
|
end
|
|
|
|
local success, result = utilities.send_message(chat_id, text, true, nil, true)
|
|
if success then
|
|
utilities.send_reply(msg, 'Your message has been sent!')
|
|
else
|
|
utilities.send_reply(msg, 'Sorry, I was unable to send your message.\n`' .. result.description .. '`', true)
|
|
end
|
|
end
|
|
|
|
return channel
|