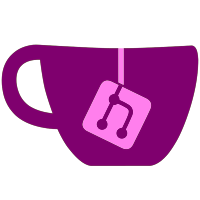
Everything reworked and rewritten. Antisquig is now a plugin to work with moderation.lua. The bot can now upload photos, stickers, and other files. Return values in plugin functions to affect the bot's behavior. All this and more!
122 lines
2.2 KiB
Lua
Executable File
122 lines
2.2 KiB
Lua
Executable File
-- utilities.lua
|
||
-- Functions shared among plugins.
|
||
|
||
function get_word(str, idx) -- get the indexed word in a string
|
||
|
||
local str = str:gsub('^%s*(.-)%s*$', '%1')
|
||
|
||
str = string.gsub(str, '\n', ' ')
|
||
if not string.find(str, ' ') then
|
||
if idx == 1 then
|
||
return str
|
||
else
|
||
return false
|
||
end
|
||
end
|
||
|
||
str = str .. ' '
|
||
if idx ~= 1 then
|
||
for i = 2, idx do
|
||
str = string.sub(str, string.find(str, ' ') + 1)
|
||
end
|
||
end
|
||
str = str:sub(1, str:find(' '))
|
||
|
||
return str:sub(1, -2)
|
||
|
||
end
|
||
|
||
function string:input() -- Returns the string after the first space.
|
||
if not self:find(' ') then
|
||
return false
|
||
end
|
||
return self:sub(self:find(' ')+1)
|
||
end
|
||
|
||
-- I swear, I copied this from PIL, not yago! :)
|
||
function string:trim() -- Trims whitespace from a string.
|
||
local s = self:gsub('^%s*(.-)%s*$', '%1')
|
||
return s
|
||
end
|
||
|
||
local lc_list = {
|
||
-- Latin = 'Cyrillic'
|
||
['A'] = 'А',
|
||
['B'] = 'В',
|
||
['C'] = 'С',
|
||
['E'] = 'Е',
|
||
['I'] = 'І',
|
||
['J'] = 'Ј',
|
||
['K'] = 'К',
|
||
['M'] = 'М',
|
||
['H'] = 'Н',
|
||
['O'] = 'О',
|
||
['P'] = 'Р',
|
||
['S'] = 'Ѕ',
|
||
['T'] = 'Т',
|
||
['X'] = 'Х',
|
||
['Y'] = 'Ү',
|
||
['a'] = 'а',
|
||
['c'] = 'с',
|
||
['e'] = 'е',
|
||
['i'] = 'і',
|
||
['j'] = 'ј',
|
||
['o'] = 'о',
|
||
['s'] = 'ѕ',
|
||
['x'] = 'х',
|
||
['y'] = 'у',
|
||
['!'] = 'ǃ'
|
||
}
|
||
|
||
function latcyr(str) -- Replaces letters with corresponding Cyrillic characters.
|
||
for k,v in pairs(lc_list) do
|
||
str = string.gsub(str, k, v)
|
||
end
|
||
return str
|
||
end
|
||
|
||
function load_data(filename) -- Loads a JSON file as a table.
|
||
|
||
local f = io.open(filename)
|
||
if not f then
|
||
return {}
|
||
end
|
||
local s = f:read('*all')
|
||
f:close()
|
||
local data = JSON.decode(s)
|
||
|
||
return data
|
||
|
||
end
|
||
|
||
function save_data(filename, data) -- Saves a table to a JSON file.
|
||
|
||
local s = JSON.encode(data)
|
||
local f = io.open(filename, 'w')
|
||
f:write(s)
|
||
f:close()
|
||
|
||
end
|
||
|
||
-- Gets coordinates for a location. Used by gMaps.lua, time.lua, weather.lua.
|
||
function get_coords(input)
|
||
|
||
local url = 'http://maps.googleapis.com/maps/api/geocode/json?address=' .. URL.escape(input)
|
||
|
||
local jstr, res = HTTP.request(url)
|
||
if res ~= 200 then
|
||
return config.errors.connection
|
||
end
|
||
|
||
local jdat = JSON.decode(jstr)
|
||
if jdat.status == 'ZERO_RESULTS' then
|
||
return config.errors.results
|
||
end
|
||
|
||
return {
|
||
lat = jdat.results[1].geometry.location.lat,
|
||
lon = jdat.results[1].geometry.location.lng
|
||
}
|
||
|
||
end
|