mirror of
https://github.com/Sude-/lgogdownloader.git
synced 2024-11-20 11:49:17 +01:00
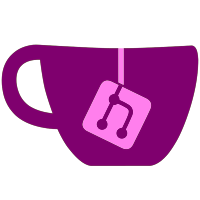
"platform" and "language" options allow using string to set them. This allows user to set them more easily without needing to calculate the sum of integer values and makes the config easier to understand later. For example: this allows setting "language" to English, German and French with "en+de+fr" which is much easier to understand than setting it to "7". Directory options can be overridden using game specific config file New options in game specific config file: - "subdirectories" - <bool> - "directory" - <string> - "subdir-game" - <string> - "subdir-installers" - <string> - "subdir-extras" - <string> - "subdir-patches" - <string> - "subdir-language-packs" - <string> - "subdir-dlc" - <string>
67 lines
2.8 KiB
C++
67 lines
2.8 KiB
C++
/* This program is free software. It comes without any warranty, to
|
|
* the extent permitted by applicable law. You can redistribute it
|
|
* and/or modify it under the terms of the Do What The Fuck You Want
|
|
* To Public License, Version 2, as published by Sam Hocevar. See
|
|
* http://www.wtfpl.net/ for more details. */
|
|
|
|
#ifndef UTIL_H
|
|
#define UTIL_H
|
|
|
|
#include "globalconstants.h"
|
|
|
|
#include <cstdio>
|
|
#include <cstdlib>
|
|
#include <cstring>
|
|
#include <cerrno>
|
|
#include <iostream>
|
|
#include <sstream>
|
|
#include <rhash.h>
|
|
#include <boost/filesystem.hpp>
|
|
#include <boost/regex.hpp>
|
|
#include <json/json.h>
|
|
|
|
struct gameSpecificDirectoryConfig
|
|
{
|
|
bool bSubDirectories;
|
|
std::string sDirectory;
|
|
std::string sGameSubdir;
|
|
std::string sInstallersSubdir;
|
|
std::string sExtrasSubdir;
|
|
std::string sPatchesSubdir;
|
|
std::string sLanguagePackSubdir;
|
|
std::string sDLCSubdir;
|
|
};
|
|
|
|
struct gameSpecificConfig
|
|
{
|
|
unsigned int iInstallerPlatform;
|
|
unsigned int iInstallerLanguage;
|
|
bool bDLC;
|
|
bool bIgnoreDLCCount;
|
|
gameSpecificDirectoryConfig dirConf;
|
|
};
|
|
|
|
namespace Util
|
|
{
|
|
std::string makeFilepath(const std::string& directory, const std::string& path, const std::string& gamename, std::string subdirectory = "", const unsigned int& platformId = 0, const std::string& dlcname = "");
|
|
std::string makeRelativeFilepath(const std::string& path, const std::string& gamename, std::string subdirectory = "");
|
|
std::string getFileHash(const std::string& filename, unsigned hash_id);
|
|
std::string getChunkHash(unsigned char* chunk, uintmax_t chunk_size, unsigned hash_id);
|
|
int createXML(std::string filepath, uintmax_t chunk_size, std::string xml_dir = std::string());
|
|
int getGameSpecificConfig(std::string gamename, gameSpecificConfig* conf, std::string directory = std::string());
|
|
int replaceString(std::string& str, const std::string& to_replace, const std::string& replace_with);
|
|
void filepathReplaceReservedStrings(std::string& str, const std::string& gamename, const unsigned int& platformId = 0, const std::string& dlcname = "");
|
|
void setFilePermissions(const boost::filesystem::path& path, const boost::filesystem::perms& permissions);
|
|
int getTerminalWidth();
|
|
void getDownloaderUrlsFromJSON(const Json::Value &root, std::vector<std::string> &urls);
|
|
std::vector<std::string> getDLCNamesFromJSON(const Json::Value &root);
|
|
std::string getHomeDir();
|
|
std::string getConfigHome();
|
|
std::string getCacheHome();
|
|
std::vector<std::string> tokenize(const std::string& str, const std::string& separator = ",");
|
|
unsigned int getOptionValue(const std::string& str, const std::vector<GlobalConstants::optionsStruct>& options);
|
|
std::string getOptionNameString(const unsigned int& value, const std::vector<GlobalConstants::optionsStruct>& options);
|
|
}
|
|
|
|
#endif // UTIL_H
|