mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
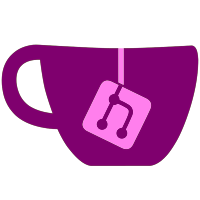
* autogen.sh : simple config for the glue build * configure.in : simple config for the glue build * makefile : add glue dir to build * glib/SList.cs : Fix some leakage. * glue/value.c : a helper function for heap alloc of GValues * glue/Makefile.am : build for libgtksharpglue svn path=/trunk/gtk-sharp/; revision=4042
65 lines
1.1 KiB
C#
65 lines
1.1 KiB
C#
// SList.cs - GSList class wrapper implementation
|
|
//
|
|
// Authors: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2002 Mike Kestner
|
|
|
|
namespace GLib {
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.Runtime.InteropServices;
|
|
|
|
/// <summary>
|
|
/// SList Class
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Wrapper class for GSList.
|
|
/// </remarks>
|
|
|
|
public class SList : ArrayList {
|
|
|
|
private IntPtr list_ptr;
|
|
|
|
[DllImport("gobject-2.0")]
|
|
static extern void g_slist_free(IntPtr l);
|
|
|
|
~SList ()
|
|
{
|
|
if (list_ptr != IntPtr.Zero)
|
|
g_slist_free (list_ptr);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Handle Property
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// A raw GSList reference for marshaling situations.
|
|
/// </remarks>
|
|
|
|
[DllImport("gobject-2.0")]
|
|
static extern IntPtr g_slist_append(IntPtr l, IntPtr d);
|
|
|
|
public IntPtr Handle {
|
|
get {
|
|
if (list_ptr != IntPtr.Zero)
|
|
g_slist_free (list_ptr);
|
|
|
|
IntPtr l = IntPtr.Zero;
|
|
foreach (object o in this) {
|
|
IntPtr data = IntPtr.Zero;
|
|
if (o is GLib.Object)
|
|
l = g_slist_append (l, ((GLib.Object)o).Handle);
|
|
else
|
|
throw new Exception();
|
|
}
|
|
|
|
list_ptr = l;
|
|
return l;
|
|
}
|
|
}
|
|
}
|
|
}
|