mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 09:05:06 +01:00
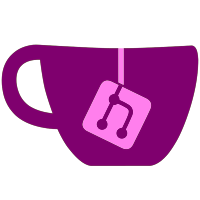
- made the following fanart changes: *fanart still auto displays *only fanart shows. no cover and no game title and no buttons (play, back, favs, etc.) *to stop and exit fanart press 'a' while pointer hand is on screen. if pointer hand is not on screen pressing 'a' launches game. pressing 'b' exits game selected screen and returns to normal coverflow. d-pad right and left still go to next or previous game. *fanart can either play once or loop. when play once is done it automatically clears fanart and returns to cover view. with looping it will restart the fanart. looping is good for slideshows of game images. looping and play once is determined by the setting "show_cover_after_animation". if set to no then looping is enabled. set to yes for only once.
91 lines
1.5 KiB
C++
91 lines
1.5 KiB
C++
// Fan Art
|
|
|
|
#ifndef __FANART_HPP
|
|
#define __FANART_HPP
|
|
|
|
#include <ogcsys.h>
|
|
#include <gccore.h>
|
|
#include <string>
|
|
|
|
#include "gui.hpp"
|
|
#include "texture.hpp"
|
|
#include "config/config.hpp"
|
|
|
|
class CFanartElement
|
|
{
|
|
public:
|
|
CFanartElement(Config &cfg, const char *dir, int artwork);
|
|
void Cleanup(void);
|
|
|
|
void draw();
|
|
void tick();
|
|
|
|
bool IsValid();
|
|
bool IsAnimationComplete();
|
|
bool ShowOnTop();
|
|
private:
|
|
TexData m_art;
|
|
int m_artwork;
|
|
int m_delay;
|
|
int m_event_duration;
|
|
|
|
int m_x;
|
|
int m_y;
|
|
int m_alpha;
|
|
float m_scaleX;
|
|
float m_scaleY;
|
|
float m_angle;
|
|
|
|
int m_event_x;
|
|
int m_event_y;
|
|
int m_event_alpha;
|
|
float m_event_scaleX;
|
|
float m_event_scaleY;
|
|
float m_event_angle;
|
|
|
|
float m_step_x;
|
|
float m_step_y;
|
|
float m_step_alpha;
|
|
float m_step_scaleX;
|
|
float m_step_scaleY;
|
|
float m_step_angle;
|
|
|
|
bool m_show_on_top;
|
|
|
|
bool m_isValid;
|
|
};
|
|
|
|
class CFanart
|
|
{
|
|
public:
|
|
CFanart(void);
|
|
~CFanart(void);
|
|
|
|
void unload();
|
|
bool load(Config &m_globalConfig, const char *path, const char *id, bool plugin_rom);
|
|
bool isAnimationComplete();
|
|
bool isLoaded();
|
|
|
|
void getBackground(const TexData * &hq, const TexData * &lq);
|
|
void draw(bool front = true);
|
|
void tick();
|
|
bool noLoop();
|
|
void reset();
|
|
|
|
private:
|
|
vector<CFanartElement> m_elms;
|
|
|
|
bool m_animationComplete;
|
|
u16 m_delayAfterAnimation;
|
|
u8 m_globalHideCover;
|
|
u8 m_globalShowCoverAfterAnimation;
|
|
u16 m_defaultDelay;
|
|
bool m_allowArtworkOnTop;
|
|
bool m_loaded;
|
|
Config m_cfg;
|
|
|
|
TexData m_bg;
|
|
TexData m_bglq;
|
|
};
|
|
|
|
#endif // __FANART_HPP
|