mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 16:38:46 +02:00
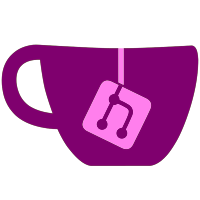
Skyline's `exception` class now stores a list of all stack frames during the invocation of the exception. These can later be parsed by the exception handler to generate a human-readable stack trace. To assist with more complete stack traces, `-fno-omit-frame-pointer` is now passed on debug builds which forces the inclusion of frames on function calls.
27 lines
926 B
C++
27 lines
926 B
C++
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright © 2022 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#pragma once
|
|
|
|
#include <vector>
|
|
#include "base.h"
|
|
|
|
namespace skyline {
|
|
/**
|
|
* @brief A wrapper over std::runtime_error with {fmt} formatting and stack tracing support
|
|
*/
|
|
class exception : public std::runtime_error {
|
|
public:
|
|
std::vector<void *> frames; //!< All frames from the stack trace during the exception
|
|
|
|
/**
|
|
* @return A vector of all frames on the caller's stack
|
|
* @note This cannot be inlined because it depends on having one stack frame itself consistently
|
|
*/
|
|
static std::vector<void*> GetStackFrames() __attribute__((noinline));
|
|
|
|
template<typename S, typename... Args>
|
|
exception(const S &formatStr, Args &&... args) : runtime_error(util::Format(formatStr, args...)), frames(GetStackFrames()) {}
|
|
};
|
|
}
|