mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 11:38:47 +02:00
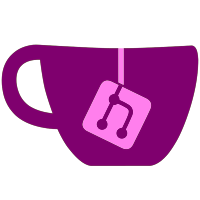
This commit mainly fixes the problem with process leakage before where the guest process wouldn't be killed. In addition, it clears up the problem with naming differences with PID/TID where purely PID was used before but that term is generally used to refer to the PGID. So, `KProcess` has a `pid` member but `KThread` has a `tid` member.
46 lines
1.9 KiB
C++
46 lines
1.9 KiB
C++
// SPDX-License-Identifier: LGPL-3.0-or-later
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#include <sys/resource.h>
|
|
#include <nce.h>
|
|
#include "KThread.h"
|
|
#include "KProcess.h"
|
|
|
|
namespace skyline::kernel::type {
|
|
KThread::KThread(const DeviceState &state, KHandle handle, pid_t selfTid, u64 entryPoint, u64 entryArg, u64 stackTop, u64 tls, u8 priority, KProcess *parent, std::shared_ptr<type::KSharedMemory> &tlsMemory)
|
|
: handle(handle), tid(selfTid), entryPoint(entryPoint), entryArg(entryArg), stackTop(stackTop), tls(tls), priority(priority), parent(parent), ctxMemory(tlsMemory), KSyncObject(state, KType::KThread) {
|
|
UpdatePriority(priority);
|
|
}
|
|
|
|
KThread::~KThread() {
|
|
Kill();
|
|
}
|
|
|
|
void KThread::Start() {
|
|
if (status == Status::Created) {
|
|
if (tid == parent->pid)
|
|
parent->status = KProcess::Status::Started;
|
|
status = Status::Running;
|
|
|
|
state.nce->StartThread(entryArg, handle, parent->threads.at(tid));
|
|
}
|
|
}
|
|
|
|
void KThread::Kill() {
|
|
if (status != Status::Dead) {
|
|
status = Status::Dead;
|
|
Signal();
|
|
tgkill(parent->pid, tid, SIGKILL);
|
|
}
|
|
}
|
|
|
|
void KThread::UpdatePriority(u8 priority) {
|
|
this->priority = priority;
|
|
auto linuxPriority =
|
|
static_cast<int8_t>(constant::AndroidPriority.first + ((static_cast<float>(constant::AndroidPriority.second - constant::AndroidPriority.first) / static_cast<float>(constant::SwitchPriority.second - constant::SwitchPriority.first)) * (static_cast<float>(priority) - constant::SwitchPriority.first))); // Resize range SwitchPriority (Nintendo Priority) to AndroidPriority (Android Priority)
|
|
|
|
if (setpriority(PRIO_PROCESS, static_cast<id_t>(tid), linuxPriority) == -1)
|
|
throw exception("Couldn't set process priority to {} for PID: {}", linuxPriority, tid);
|
|
}
|
|
}
|