mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 15:48:47 +02:00
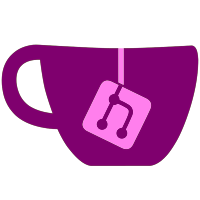
Certain titles depend on HID LIFO entries being written out at a fixed frequency rather than on actual state change, not doing this can lead to applications freezing till the LIFO is filled up to maximum size, this behavior is seen in Super Mario Odyssey. In other cases such as Metroid Dread, the game can run into race conditions that would lead to crashes, these were worked around by smashing a button during loading prior. This commit introduces a thread which sleeps and wakes up occasionally to write LIFO entries into HID shared memory at the desired frequencies. This alleviates any issues as it fills up the LIFO instantly and correctly emulates HID Shared Memory behavior expected by the guest. Co-authored-by: Narr the Reg <juangerman-13@hotmail.com>
38 lines
1.1 KiB
C++
38 lines
1.1 KiB
C++
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#pragma once
|
|
|
|
#include "common.h"
|
|
#include "kernel/types/KSharedMemory.h"
|
|
#include "input/shared_mem.h"
|
|
#include "input/npad.h"
|
|
#include "input/touch.h"
|
|
|
|
namespace skyline::input {
|
|
/**
|
|
* @brief The Input class manages components responsible for translating host input to guest input
|
|
*/
|
|
class Input {
|
|
private:
|
|
const DeviceState &state;
|
|
|
|
public:
|
|
std::shared_ptr<kernel::type::KSharedMemory> kHid; //!< The kernel shared memory object for HID Shared Memory
|
|
HidSharedMemory *hid; //!< A pointer to HID Shared Memory on the host
|
|
|
|
NpadManager npad;
|
|
TouchManager touch;
|
|
|
|
Input(const DeviceState &state);
|
|
|
|
private:
|
|
std::thread updateThread; //!< A thread that handles delivering HID shared memory updates at a fixed rate
|
|
|
|
/**
|
|
* @brief The entry point for the update thread, this handles timing and delegation to the shared memory managers
|
|
*/
|
|
void UpdateThread();
|
|
};
|
|
}
|