mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-07-11 20:31:49 +02:00
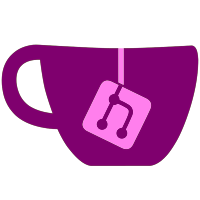
new vars are firstLine = 1; numLines = -1; totalLines = 1; totalLines is not changed until the text is set to wrap and Draw() is called 1 time. The other 2 are set with functions. new functions are void GuiText::SetNumLines(int n) void GuiText::SetFirstLine(int n) int GuiText::GetNumLines() int GuiText::GetFirstLine() int GuiText::GetTotalLines() int GuiText::GetLineHeight(int n) These should be self explanatory. GetLineHeight relies on total lines so it needs to be called after the first Draw(); Raised the chars[] to 3000 for the synopsis because people are writing books in there. Used those new fancy functions make the synopsis fit in the info window. Press up/down to scroll the text. Arrows may come later for the buttons. Also put a home buttononlytrigger on the game prompt. moved disable mainWindow to the beginning of the infoprompt to keep from loading images and stuff from the sd at the same time we are reading the xml file.
79 lines
1.8 KiB
C
79 lines
1.8 KiB
C
|
|
#ifndef _XML_H_
|
|
#define _XML_H_
|
|
|
|
|
|
#ifdef __cplusplus
|
|
extern "C"
|
|
{
|
|
#endif
|
|
|
|
// open database, close database, load info for a game
|
|
bool OpenXMLDatabase(char* xmlfilepath, char* argdblang, bool argJPtoEN, bool openfile, bool loadtitles, bool keepopen);
|
|
void CloseXMLDatabase();
|
|
bool LoadGameInfoFromXML(char* gameid, char* langcode);
|
|
|
|
|
|
#define XML_ELEMMAX 15
|
|
|
|
struct gameXMLinfo
|
|
{
|
|
char id[7];
|
|
char version[50];
|
|
char region[7];
|
|
char title[100];
|
|
char synopsis[3000];
|
|
char title_EN[100];
|
|
char synopsis_EN[3000];
|
|
char locales[XML_ELEMMAX+1][3];
|
|
int localeCnt;
|
|
char developer[75];
|
|
char publisher[75];
|
|
char publisherfromid[75];
|
|
char year[5];
|
|
char month[3];
|
|
char day[3];
|
|
char genre[75];
|
|
char genresplit[XML_ELEMMAX+1][20];
|
|
int genreCnt;
|
|
char ratingtype[5];
|
|
char ratingvalue[5];
|
|
char ratingdescriptors[XML_ELEMMAX+1][40];
|
|
int descriptorCnt;
|
|
char ratingvalueCERO[5];
|
|
char ratingvalueESRB[5];
|
|
char ratingvaluePEGI[5];
|
|
char wifiplayers[4];
|
|
char wififeatures[XML_ELEMMAX+1][20];
|
|
int wifiCnt;
|
|
char players[4];
|
|
char accessories[XML_ELEMMAX+1][20];
|
|
int accessoryCnt;
|
|
char accessoriesReq[XML_ELEMMAX+1][20];
|
|
int accessoryReqCnt;
|
|
char iso_crc[9];
|
|
char iso_md5[33];
|
|
char iso_sha1[41];
|
|
} ;
|
|
|
|
struct gameXMLinfo gameinfo;
|
|
struct gameXMLinfo gameinfo_reset;
|
|
|
|
bool OpenXMLFile(char* filename);
|
|
void LoadTitlesFromXML(char *langcode, bool forcejptoen);
|
|
void GetPublisherFromGameid(char *idtxt, char *dest, int destsize);
|
|
char *ConvertLangTextToCode(char *langtext);
|
|
void ConvertRating(char *ratingvalue, char *fromrating, char *torating, char *destvalue, int destsize);
|
|
void PrintGameInfo(bool showfullinfo);
|
|
char *MemInfo();
|
|
|
|
void title_set(char *id, char *title);
|
|
char* trimcopy(char *dest, char *src, int size);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif
|
|
|