mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-11-05 02:55:07 +01:00
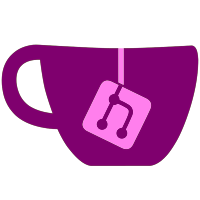
*added a temporary workaround for selecting favorites when no favorites were setup for grid and carousel layout because of some serious problem in there which i have to look for later. *Reworked complete cover/discart download function (seems a lot faster to me now) *Fixed problem with switching games in game prompt and then going to the game settings. Was always the first selected games settings. *Fixed crash when clicking the DVD icon *Fixed save of lock/unlock state in parental control *Fixed install menu messup (not return to right menus) *Removed unnecessary save of settings on every mode switch *Several cleanups and fixes *Removed mountMethod 3
61 lines
1.9 KiB
C++
61 lines
1.9 KiB
C++
#include <stdio.h>
|
|
#include <string>
|
|
#include <vector>
|
|
#include "FileOperations/fileops.h"
|
|
#include "usbloader/GameList.h"
|
|
#include "wstring.hpp"
|
|
#include "gecko.h"
|
|
|
|
/**************************************************************************************
|
|
* FindMissingFiles
|
|
* Inputs:
|
|
* path - Path to search in with. example "SD:/covers/"
|
|
* fileext - the file extension. example ".png"
|
|
* MissingFilesList - string vector where the IDs of missing game files will be put in.
|
|
**************************************************************************************/
|
|
int GetMissingGameFiles(const char * path, const char * fileext, std::vector<std::string> & MissingFilesList)
|
|
{
|
|
char gameID[7];
|
|
char filepath[512];
|
|
MissingFilesList.clear();
|
|
wString oldFilter(gameList.GetCurrentFilter());
|
|
|
|
//! make sure that all games are added to the gamelist
|
|
gameList.LoadUnfiltered();
|
|
|
|
for (int i = 0; i < gameList.size(); ++i)
|
|
{
|
|
struct discHdr* header = gameList[i];
|
|
snprintf(gameID, sizeof(gameID), "%s", (char *) header->id);
|
|
snprintf(filepath, sizeof(filepath), "%s/%s%s", path, gameID, fileext);
|
|
|
|
if (CheckFile(filepath))
|
|
continue;
|
|
|
|
//! Not found. Try 4 ID path.
|
|
gameID[4] = '\0';
|
|
snprintf(filepath, sizeof(filepath), "%s/%s%s", path, gameID, fileext);
|
|
|
|
if (CheckFile(filepath))
|
|
continue;
|
|
|
|
//! Not found. Try 3 ID path.
|
|
gameID[3] = '\0';
|
|
snprintf(filepath, sizeof(filepath), "%s/%s%s", path, gameID, fileext);
|
|
|
|
if (CheckFile(filepath))
|
|
continue;
|
|
|
|
//! Not found add to missing list
|
|
snprintf(gameID, sizeof(gameID), "%s", (char *) header->id);
|
|
MissingFilesList.push_back(std::string(gameID));
|
|
}
|
|
|
|
//! Bring game list to the old state
|
|
gameList.FilterList(oldFilter.c_str());
|
|
|
|
gprintf(" = %i", MissingFilesList.size());
|
|
|
|
return MissingFilesList.size();
|
|
}
|