mirror of
https://github.com/wiidev/usbloadergx.git
synced 2024-06-29 22:46:07 +02:00
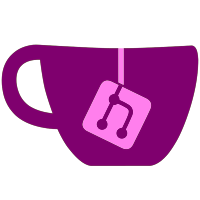
*Fix crash in cheat menu when the browser has an empty entry *Improved detection of not existing cheat txt files (404 Page Not Found) *Added a per game save game extraction to emu nand (right now only from /title/00010000/, others will come when users report which are needed for which games :P) *Added feature to extract all save games to emu nand *Added proper message to install progress bar to show when install is really finished and a game list reload starts (wait time is taking too long sometimes) *Fixed a little bug in the progress bar *Several code optimizations *Changed default make file build to IOS249 build since most people use d2x now
47 lines
1.0 KiB
C++
47 lines
1.0 KiB
C++
#ifndef _GUIGAMELIST_H_
|
|
#define _GUIGAMELIST_H_
|
|
|
|
#include "gui_gamebrowser.h"
|
|
#include "gui_scrollbar.hpp"
|
|
#include "usbloader/disc.h"
|
|
|
|
class GuiGameList : public GuiGameBrowser, public sigslot::has_slots<>
|
|
{
|
|
public:
|
|
GuiGameList(int w, int h, int listOffset = 0);
|
|
virtual ~GuiGameList();
|
|
int GetClickedOption();
|
|
int GetSelectedOption() { return listOffset+selectedItem; }
|
|
void SetSelectedOption(int ind);
|
|
void setListOffset(int off);
|
|
int getListOffset() const { return listOffset; }
|
|
void ResetState();
|
|
void SetFocus(int f);
|
|
void Draw();
|
|
void Update(GuiTrigger * t);
|
|
protected:
|
|
void onListChange(int SelItem, int SelInd);
|
|
void UpdateListEntries();
|
|
int selectedItem;
|
|
int listOffset;
|
|
int pagesize;
|
|
int maxTextWidth;
|
|
|
|
GuiButton ** game;
|
|
GuiText ** gameTxt;
|
|
GuiText ** gameTxtOver;
|
|
GuiImage ** gameBg;
|
|
GuiImage ** newImg;
|
|
|
|
GuiImage * bgGameImg;
|
|
|
|
GuiImageData * bgGames;
|
|
GuiImageData * bgGamesEntry;
|
|
GuiImageData * newGames;
|
|
|
|
GuiTrigger * trigA;
|
|
|
|
GuiScrollbar scrollBar;
|
|
};
|
|
#endif
|